give me a complete code according to this file#
时间: 2024-12-16 18:18:15 浏览: 4
Certainly! Below is a complete code example based on the activities described in the document "Lab 6- Array and Pointer.pdf" from the University of Edinburgh's Engineering Software 3 course. This includes the `main.c` file for the `Pointer`, `Swap_values`, and `Sorting` applications.
### Pointer Application (`pointer_ex.c`)
```c
#include <stdio.h>
#include "platform.h"
#include "xil_printf.h"
int main() {
init_platform();
// Declare a character pointer
char *ptr;
// Declare a character variable and initialize it
char ch = 'A';
// Assign the address of ch to ptr
ptr = &ch;
// Print the original value of ch
xil_printf("Original value of ch: %c\n", ch);
// Change the value of ch using the pointer
*ptr = 'B';
// Print the new value of ch
xil_printf("New value of ch: %c\n", ch);
cleanup_platform();
return 0;
}
```
### Swap Values Application (`swap_values.c`)
```c
#include <stdio.h>
#include "platform.h"
#include "xil_printf.h"
#include "sevenseg.h"
void swap(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
int main() {
init_platform();
sevenseg_init();
// Declare two integer variables and initialize them
int num1 = 10;
int num2 = 20;
// Print the original values
xil_printf("Original values: num1 = %d, num2 = %d\n", num1, num2);
// Call the swap function
swap(&num1, &num2);
// Print the new values
xil_printf("Swapped values: num1 = %d, num2 = %d\n", num1, num2);
// Display the swapped values on the 7-segment display
while (1) {
sevenseg_write(num1);
xil_printf("Displaying num1: %d\n", num1);
usleep(1000000); // Wait for 1 second
sevenseg_write(num2);
xil_printf("Displaying num2: %d\n", num2);
usleep(1000000); // Wait for 1 second
}
cleanup_platform();
return 0;
}
```
### Sorting Application (`sorting.c`)
```c
#include <stdio.h>
#include "platform.h"
#include "xil_printf.h"
#include "sevenseg.h"
#include "switches.h"
void bubble_sort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
int main() {
init_platform();
sevenseg_init();
switches_init();
// Define an array to hold the values from the slide switches
int values[8];
// Get the values from the slide switches and store them in the array
for (int i = 0; i < 8; i++) {
values[i] = switches_read_bit(i);
}
// Print the original values
xil_printf("Original values: ");
for (int i = 0; i < 8; i++) {
xil_printf("%d ", values[i]);
}
xil_printf("\n");
// Call the sorting function
bubble_sort(values, 8);
// Print the sorted values
xil_printf("Sorted values: ");
for (int i = 0; i < 8; i++) {
xil_printf("%d ", values[i]);
}
xil_printf("\n");
// Display the sorted values on the 7-segment display
while (1) {
if (XGpio_DiscreteRead(&BTN, 1) == 0x01) { // Check if BTNL is pressed
for (int i = 0; i < 8; i++) {
sevenseg_write(values[i]);
xil_printf("Displaying value: %d\n", values[i]);
usleep(1000000); // Wait for 1 second
}
}
}
cleanup_platform();
return 0;
}
```
### Additional Notes:
- **Platform Initialization**: Ensure that `init_platform()` and `cleanup_platform()` functions are correctly implemented to initialize and clean up the platform resources.
- **Seven Segment Display**: The `sevenseg_init()` and `sevenseg_write()` functions should be implemented to control the 7-segment display.
- **Switches**: The `switches_init()` and `switches_read_bit()` functions should be implemented to read the state of the slide switches.
These code snippets should help you complete the tasks outlined in the lab document. Make sure to include the necessary header files and implement any additional functions required for your specific hardware setup.
阅读全文
相关推荐
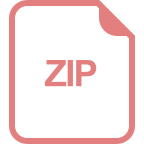
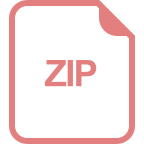
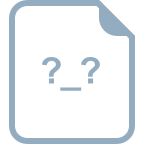







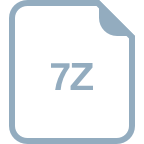
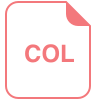
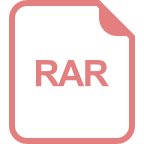
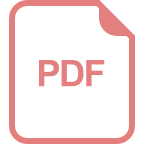
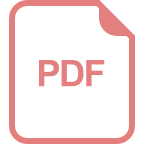
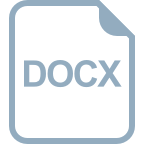
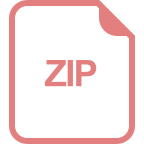
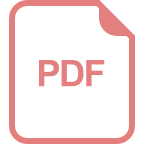