give me a complete verilog code according this file
时间: 2024-12-16 12:18:25 浏览: 5
Certainly! Below is a complete Verilog code for the VGA interface module and the wrapper module based on the provided document.
### VGA Interface Module (`VGA.v`)
```verilog
module VGA (
input wire CLK,
input wire [11:0] COLOUR_IN,
output reg [11:0] COLOUR_OUT,
output reg HS,
output reg VS,
output reg [9:0] X,
output reg [9:0] Y
);
// Parameters for synchronization signals
parameter H_FRONT_PORCH = 16;
parameter H_SYNC_WIDTH = 96;
parameter H_BACK_PORCH = 48;
parameter H_DISPLAY_TIME = 640;
parameter V_FRONT_PORCH = 10;
parameter V_SYNC_WIDTH = 2;
parameter V_BACK_PORCH = 33;
parameter V_DISPLAY_TIME = 480;
// Internal counters
reg [9:0] h_counter;
reg [9:0] v_counter;
// Always block for horizontal counter
always @(posedge CLK) begin
if (h_counter == 799) begin
h_counter <= 0;
v_counter <= v_counter + 1;
end else begin
h_counter <= h_counter + 1;
end
end
// Always block for vertical counter
always @(posedge CLK) begin
if (v_counter == 520) begin
v_counter <= 0;
end
end
// Horizontal sync signal
assign HS = (h_counter < H_FRONT_PORCH + H_SYNC_WIDTH) ? 1'b0 : 1'b1;
// Vertical sync signal
assign VS = (v_counter < V_FRONT_PORCH + V_SYNC_WIDTH) ? 1'b0 : 1'b1;
// Determine valid display region
reg valid_display;
assign valid_display = (h_counter >= H_FRONT_PORCH + H_SYNC_WIDTH + H_BACK_PORCH && h_counter < H_FRONT_PORCH + H_SYNC_WIDTH + H_BACK_PORCH + H_DISPLAY_TIME) &&
(v_counter >= V_FRONT_PORCH + V_SYNC_WIDTH + V_BACK_PORCH && v_counter < V_FRONT_PORCH + V_SYNC_WIDTH + V_BACK_PORCH + V_DISPLAY_TIME);
// Output color signal
always @(posedge CLK) begin
if (valid_display) begin
COLOUR_OUT <= COLOUR_IN;
end else begin
COLOUR_OUT <= 12'b0;
end
end
// Output X and Y coordinates
always @(posedge CLK) begin
if (valid_display) begin
X <= h_counter - (H_FRONT_PORCH + H_SYNC_WIDTH + H_BACK_PORCH);
Y <= v_counter - (V_FRONT_PORCH + V_SYNC_WIDTH + V_BACK_PORCH);
end else begin
X <= 10'b0;
Y <= 10'b0;
end
end
endmodule
```
### Wrapper Module (`VGATest.v`)
```verilog
module VGATest (
input wire CLK,
input wire [11:0] SW, // Slide switches
output reg [11:0] COLOUR_OUT,
output reg HS,
output reg VS,
output reg [9:0] X,
output reg [9:0] Y
);
// Instantiate the VGA module
VGA uut (
.CLK(CLK),
.COLOUR_IN(COLOUR_IN),
.COLOUR_OUT(COLOUR_OUT),
.HS(HS),
.VS(VS),
.X(X),
.Y(Y)
);
// Local variables
reg [11:0] COLOUR_IN;
// Always block to handle COLOUR_IN signal
always @(posedge CLK) begin
// Default color from slide switches
COLOUR_IN <= SW;
// Invert color for 10x10 pixel areas in all four corners
if ((X < 10 && Y < 10) || (X > 630 && Y < 10) || (X < 10 && Y > 470) || (X > 630 && Y > 470)) begin
COLOUR_IN <= ~SW;
end
end
endmodule
```
### Constraints File (`VGA.xdc`)
```tcl
# VGA Pin Mappings
set_property PACKAGE_PIN E3 [get_ports {CLK}]
set_property IOSTANDARD LVCMOS33 [get_ports {CLK}]
set_property PACKAGE_PIN G19 [get_ports {HS}]
set_property IOSTANDARD LVCMOS33 [get_ports {HS}]
set_property PACKAGE_PIN J19 [get_ports {VS}]
set_property IOSTANDARD LVCMOS33 [get_ports {VS}]
set_property PACKAGE_PIN L14 [get_ports {X[0]}]
set_property PACKAGE_PIN K14 [get_ports {X[1]}]
set_property PACKAGE_PIN J13 [get_ports {X[2]}]
set_property PACKAGE_PIN H13 [get_ports {X[3]}]
set_property PACKAGE_PIN N15 [get_ports {X[4]}]
set_property PACKAGE_PIN M13 [get_ports {X[5]}]
set_property PACKAGE_PIN P15 [get_ports {X[6]}]
set_property PACKAGE_PIN R15 [get_ports {X[7]}]
set_property PACKAGE_PIN T14 [get_ports {X[8]}]
set_property PACKAGE_PIN T15 [get_ports {X[9]}]
set_property PACKAGE_PIN M14 [get_ports {Y[0]}]
set_property PACKAGE_PIN M15 [get_ports {Y[1]}]
set_property PACKAGE_PIN N14 [get_ports {Y[2]}]
set_property PACKAGE_PIN P14 [get_ports {Y[3]}]
set_property PACKAGE_PIN R14 [get_ports {Y[4]}]
set_property PACKAGE_PIN T16 [get_ports {Y[5]}]
set_property PACKAGE_PIN U15 [get_ports {Y[6]}]
set_property PACKAGE_PIN U16 [get_ports {Y[7]}]
set_property PACKAGE_PIN V15 [get_ports {Y[8]}]
set_property PACKAGE_PIN W15 [get_ports {Y[9]}]
set_property PACKAGE_PIN W13 [get_ports {COLOUR_OUT[0]}]
set_property PACKAGE_PIN V13 [get_ports {COLOUR_OUT[1]}]
set_property PACKAGE_PIN W12 [get_ports {COLOUR_OUT[2]}]
set_property PACKAGE_PIN V12 [get_ports {COLOUR_OUT[3]}]
set_property PACKAGE_PIN W11 [get_ports {COLOUR_OUT[4]}]
set_property PACKAGE_PIN V11 [get_ports {COLOUR_OUT[5]}]
set_property PACKAGE_PIN W10 [get_ports {COLOUR_OUT[6]}]
set_property PACKAGE_PIN V10 [get_ports {COLOUR_OUT[7]}]
set_property PACKAGE_PIN W9 [get_ports {COLOUR_OUT[8]}]
set_property PACKAGE_PIN V9 [get_ports {COLOUR_OUT[9]}]
set_property PACKAGE_PIN W8 [get_ports {COLOUR_OUT[10]}]
set_property PACKAGE_PIN V8 [get_ports {COLOUR_OUT[11]}]
# Slide Switches
set_property PACKAGE_PIN W7 [get_ports {SW[0]}]
set_property PACKAGE_PIN V7 [get_ports {SW[1]}]
set_property PACKAGE_PIN W6 [get_ports {SW[2]}]
set_property PACKAGE_PIN V6 [get_ports {SW[3]}]
set_property PACKAGE_PIN W5 [get_ports {SW[4]}]
set_property PACKAGE_PIN V5 [get_ports {SW[5]}]
set_property PACKAGE_PIN W4 [get_ports {SW[6]}]
set_property PACKAGE_PIN V4 [get_ports {SW[7]}]
set_property PACKAGE_PIN W3 [get_ports {SW[8]}]
set_property PACKAGE_PIN V3 [get_ports {SW[9]}]
set_property PACKAGE_PIN W2 [get_ports {SW[10]}]
set_property PACKAGE_PIN V2 [get_ports {SW[11]}]
```
### Explanation
1. **VGA Interface Module (`VGA.v`)**:
- Implements the VGA interface with horizontal and vertical counters.
- Generates horizontal and vertical sync signals (`HS`, `VS`).
- Determines the valid display region and outputs the appropriate color (`COLOUR_OUT`).
- Provides the X and Y coordinates of the current pixel.
2. **Wrapper Module (`VGATest.v`)**:
- Instantiates the VGA module.
- Controls the `COLOUR_IN` signal based on the slide switches.
- Inverts the color for 10x10 pixel areas in all four corners of the display.
3. **Constraints File (`VGA.xdc`)**:
- Maps the FPGA pins to the corresponding VGA signals and slide switches.
This setup should allow you to connect your BASYS 3 board to a monitor and display colors controlled by the slide switches, with inverted colors in the specified regions.
阅读全文
相关推荐
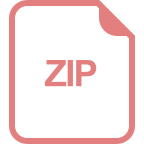















