opengl创建一个包含三维点云顶点和三维三角面片网格的数据结构,用assimp读入.ply格式的三角网格,来填充这个数据结构,将他送进渲染缓冲区完整代码
时间: 2024-09-13 12:02:13 浏览: 59
在OpenGL中创建一个包含三维点云顶点和三维三角面片网格的数据结构,通常涉及到定义合适的缓冲区来存储顶点数据和索引数据。然后,使用Assimp库来读取PLY格式的文件,填充这些数据结构。以下是一个简化的示例代码,展示如何使用OpenGL和Assimp库来完成这个任务。
请注意,这个示例假设你已经有了一个基本的OpenGL环境设置,熟悉OpenGL的基本操作,并且已经配置了Assimp库。
```c
#include <GL/glew.h>
#include <assimp/Importer.hpp>
#include <assimp/scene.h>
#include <assimp/postprocess.h>
#include <vector>
#include <iostream>
// 假设顶点结构包含位置、法线等信息
struct Vertex {
GLfloat x, y, z;
GLfloat nx, ny, nz;
// 可以添加更多属性,比如纹理坐标、颜色等
};
// 顶点缓冲对象(VBO)和索引缓冲对象(IBO)的ID
GLuint vboID, iboID, vaoID;
// 加载PLY文件并填充顶点和索引数据
bool LoadPLY(const std::string& filename, std::vector<Vertex>& vertices, std::vector<GLuint>& indices) {
Assimp::Importer importer;
const aiScene* scene = importer.ReadFile(filename, aiProcess_Triangulate | aiProcess_FlipUVs);
if (!scene || scene->mFlags & AI_SCENE_FLAGS_INCOMPLETE || !scene->mRootNode) {
std::cerr << "ERROR::ASSIMP::" << importer.GetErrorString() << std::endl;
return false;
}
// 假设模型中只有一个网格
if (scene->mNumMeshes > 0) {
aiMesh* mesh = scene->mMeshes[0];
for (unsigned int i = 0; i < mesh->mNumVertices; i++) {
Vertex v;
v.x = mesh->mVertices[i].x;
v.y = mesh->mVertices[i].y;
v.z = mesh->mVertices[i].z;
v.nx = mesh->mNormals[i].x;
v.ny = mesh->mNormals[i].y;
v.nz = mesh->mNormals[i].z;
// 添加其他属性
// ...
vertices.push_back(v);
}
for (unsigned int i = 0; i < mesh->mNumFaces; i++) {
aiFace face = mesh->mFaces[i];
for (unsigned int j = 0; j < face.mNumIndices; j++) {
indices.push_back(face.mIndices[j]);
}
}
}
return true;
}
// 初始化OpenGL缓冲区并绘制模型
void DrawModel(const std::vector<Vertex>& vertices, const std::vector<GLuint>& indices) {
// 创建并绑定VBO
glGenBuffers(1, &vboID);
glBindBuffer(GL_ARRAY_BUFFER, vboID);
glBufferData(GL_ARRAY_BUFFER, vertices.size() * sizeof(Vertex), &vertices[0], GL_STATIC_DRAW);
// 创建并绑定IBO
glGenBuffers(1, &iboID);
glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, iboID);
glBufferData(GL_ELEMENT_ARRAY_BUFFER, indices.size() * sizeof(GLuint), &indices[0], GL_STATIC_DRAW);
// 创建并绑定VAO
glGenVertexArrays(1, &vaoID);
glBindVertexArray(vaoID);
// 设置顶点属性指针
glEnableVertexAttribArray(0); // 位置
glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE, sizeof(Vertex), (GLvoid*)0);
glEnableVertexAttribArray(1); // 法线
glVertexAttribPointer(1, 3, GL_FLOAT, GL_FALSE, sizeof(Vertex), (GLvoid*)(sizeof(GLfloat) * 3));
// 绘制三角形网格
glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, iboID);
glDrawElements(GL_TRIANGLES, indices.size(), GL_UNSIGNED_INT, 0);
// 解绑VAO、VBO和IBO
glBindVertexArray(0);
glBindBuffer(GL_ARRAY_BUFFER, 0);
glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, 0);
}
int main() {
// 初始化OpenGL环境...
std::vector<Vertex> vertices;
std::vector<GLuint> indices;
if (LoadPLY("model.ply", vertices, indices)) {
DrawModel(vertices, indices);
}
// 清理OpenGL资源...
glDeleteVertexArrays(1, &vaoID);
glDeleteBuffers(1, &vboID);
glDeleteBuffers(1, &iboID);
return 0;
}
```
阅读全文
相关推荐
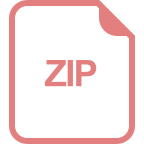
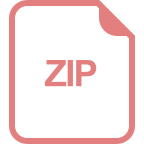

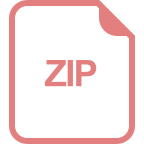
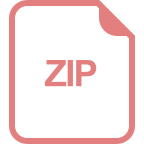
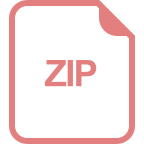
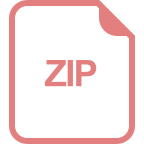
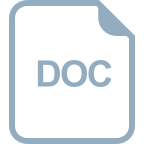
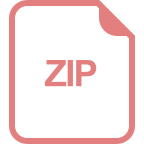
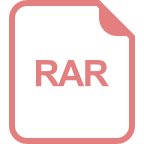
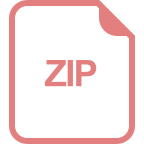
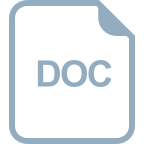




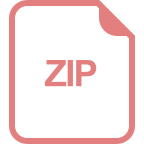