write a c++ program for the game rock paper scissor
时间: 2024-10-10 07:17:33 浏览: 36
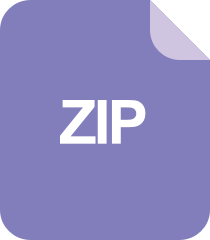
rock-paper-scissor:Rock,Paper和Scissor的简单JavaScript游戏
在C++编写一个简单的"石头、剪刀、布"游戏程序,你可以创建一个控制台应用程序,让玩家输入选择,并与电脑随机选择进行比较。以下是基本的步骤:
```cpp
#include <iostream>
#include <ctime>
#include <cstdlib>
enum Choices { ROCK, PAPER, SCISSORS };
int getComputerChoice() {
srand(time(0));
int choices[] = {ROCK, PAPER, SCISSORS};
return choices[rand() % 3];
}
void playGame() {
std::cout << "Choose your move (rock, paper, scissors): ";
char userChoice;
std::cin >> userChoice;
if (userChoice == 'r') {
userChoice = ROCK;
} else if (userChoice == 'p') {
userChoice = PAPER;
} else if (userChoice == 's') {
userChoice = SCISSORS;
} else {
std::cout << "Invalid choice! Please try again.\n";
return playGame();
}
int computerChoice = getComputerChoice();
switch (computerChoice) {
case ROCK:
if (userChoice == PAPER) {
std::cout << "You chose " << static_cast<char>(userChoice) << ", computer chose " << static_cast<char>(ROCK)
<< ". You win!\n";
} else if (userChoice == SCISSORS) {
std::cout << "You chose " << static_cast<char>(userChoice) << ", computer chose " << static_cast<char>(ROCK)
<< ". Computer wins!\n";
} else {
std::cout << "It's a tie!\n";
}
break;
case PAPER:
if (userChoice == ROCK) {
std::cout << "You chose " << static_cast<char>(userChoice) << ", computer chose " << static_cast<char>(PAPER)
<< ". Computer wins!\n";
} else if (userChoice == SCISSORS) {
std::cout << "You chose " << static_cast<char>(userChoice) << ", computer chose " << static_cast<char>(PAPER)
<< ". You win!\n";
} else {
std::cout << "It's a tie!\n";
}
break;
case SCISSORS:
if (userChoice == ROCK) {
std::cout << "You chose " << static_cast<char>(userChoice) << ", computer chose " << static_cast<char>(SCISSORS)
<< ". You lose!\n";
} else if (userChoice == PAPER) {
std::cout << "You chose " << static_cast<char>(userChoice) << ", computer chose " << static_cast<char>(SCISSORS)
<< ". You win!\n";
} else {
std::cout << "It's a tie!\n";
}
break;
}
}
int main() {
while (true) {
playGame();
std::cout << "Do you want to play again? (y/n): ";
char response;
std::cin >> response;
if (response != 'y' && response != 'Y') {
break;
}
}
return 0;
}
```
阅读全文
相关推荐
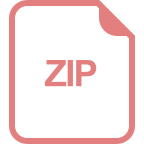
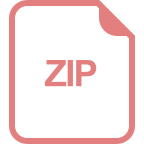

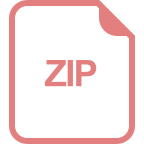
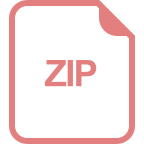
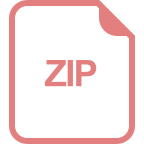
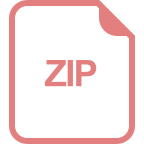
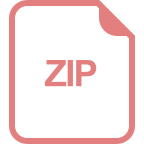
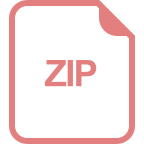
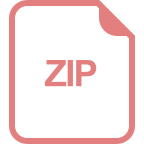
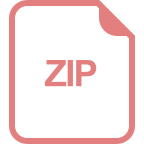
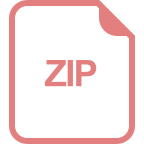
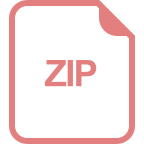

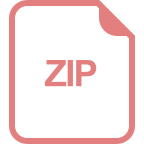
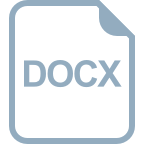