用栈实现非递归迷宫问题算法,C语言,从1,1到m,n
时间: 2024-12-15 11:17:01 浏览: 8
用栈(Stack)实现非递归的迷宫问题算法通常涉及到宽度优先搜索(Breadth-First Search, BFS)。这种算法可以用于查找从起点到终点的最短路径。以下是使用C语言的一个基本思路:
```c
#include <stdio.h>
#include <stdlib.h>
#define ROWS 5 // 迷宫的行数
#define COLS 5 // 迷宫的列数
// 检查当前位置是否有效
int isValid(int x, int y) {
return (x >= 0 && x < ROWS && y >= 0 && y < COLS && maze[x][y] == 0);
}
// 动态数组表示栈
typedef struct Stack {
int arr[ROWS * COLS];
int top;
} Stack;
// 初始化栈
void init(Stack *s) {
s->top = -1;
}
// 入栈操作
void push(Stack *s, int pos) {
if (s->top < ROWS * COLS - 1)
s->arr[++(s->top)] = pos;
}
// 出栈操作
int pop(Stack *s) {
if (s->top == -1)
return -1; // 空栈
return s->arr[s->top--];
}
// 栈顶元素
int peek(Stack *s) {
return s->arr[s->top];
}
// 非递归迷宫算法
void mazeSolver(int maze[][COLS], int startRow, int startCol, int endRow, int endCol, Stack *stack) {
stack->top = -1;
init(stack);
// 将起始位置入栈
push(stack, startRow * COLS + startCol);
maze[startRow][startCol] = 1; // 标记为已访问
while (stack->top != -1) {
int currentPos = pop(stack); // 取出下一个位置
int row = currentPos / COLS;
int col = currentPos % COLS;
// 如果到达了终点,结束搜索
if (row == endRow && col == endCol) {
printf("找到了一条路径:\n");
printPath(maze, row, col, startRow, startCol);
break;
}
// 检查周围未访问过的相邻节点
for (int dr = -1; dr <= 1; dr++) {
for (int dc = -1; dc <= 1; dc++) {
int newRow = row + dr;
int newCol = col + dc;
if (isValid(newRow, newCol)) {
if (maze[newRow][newCol] == 0) {
printf("(%d,%d)->", newRow, newCol);
push(stack, newRow * COLS + newCol);
maze[newRow][newCol] = 1; // 标记为已访问
}
}
}
}
}
}
// 打印路径
void printPath(int maze[][COLS], int endRow, int endCol, int startRow, int startCol) {
int path[endRow * COLS + endCol];
int i = 0;
while (endRow != startRow || endCol != startCol) {
path[i++] = maze[endRow][endCol];
if (endRow > startRow) {
endRow--;
} else {
endCol++;
}
}
printf("(%d,%d)\n", startRow, startCol);
for (i--; i >= 0; i--)
printf("%d ", path[i]);
printf("\n");
}
int main() {
int maze[ROWS][COLS] = { // 你的迷宫矩阵
{1, 1, 1, 1, 0},
{1, 0, 0, 0, 1},
{1, 0, 1, 1, 1},
{1, 0, 0, 0, 1},
{1, 1, 1, 1, 1}
};
mazeSolver(maze, 0, 0, ROWS - 1, COLS - 1, &stack);
return 0;
}
```
这个程序首先初始化栈,并将起点放入栈中。然后在每次循环中,它会取出栈顶位置并检查其周围的邻居。如果邻居尚未访问并且是空格(0),则标记为已访问并将坐标压入栈中。当找到终点时,回溯路径并打印出来。
阅读全文
相关推荐







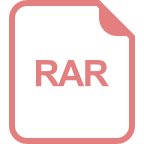
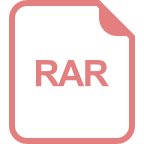








