thrift python
时间: 2023-08-26 22:13:08 浏览: 150
Thrift is a framework for building cross-language services. It allows you to define data types and service interfaces in a simple definition file, and then generate code in various languages to implement those interfaces. In Python, you can use the Thrift library to create both client and server applications.
To use Thrift in Python, you first need to install the Thrift library. You can do this using pip:
```
pip install thrift
```
Once installed, you can start using Thrift in your Python code. Here's a simple example to get you started:
1. Define your data types and service interface in a Thrift IDL file (e.g., `example.thrift`):
```
namespace py example
struct MyStruct {
1: required string name
2: optional i32 age
}
service MyService {
MyStruct getStruct(1: string id)
}
```
2. Generate Python code from the IDL file using the `thrift` compiler:
```
thrift --gen py example.thrift
```
3. Implement the service interface in Python:
```python
from example import MyService, ttypes
class MyHandler:
def getStruct(self, id):
# Implementation code goes here
return ttypes.MyStruct(name="John", age=25)
handler = MyHandler()
processor = MyService.Processor(handler)
# Run the server
transport = TSocket.TServerSocket(port=9090)
tfactory = TTransport.TBufferedTransportFactory()
pfactory = TBinaryProtocol.TBinaryProtocolFactory()
server = TServer.TSimpleServer(processor, transport, tfactory, pfactory)
server.serve()
```
4. Create a client to interact with the server:
```python
from example import MyService, ttypes
transport = TSocket.TSocket("localhost", 9090)
transport = TTransport.TBufferedTransport(transport)
protocol = TBinaryProtocol.TBinaryProtocol(transport)
client = MyService.Client(protocol)
transport.open()
struct = client.getStruct("123")
print(struct.name)
print(struct.age)
transport.close()
```
This is just a basic example to give you an idea of how to use Thrift with Python. You can find more details and advanced usage in the Thrift documentation.
阅读全文
相关推荐
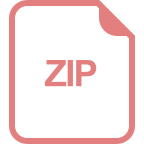
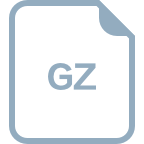
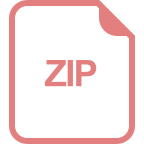















