pandas 如何判断str内开头是否有~$
时间: 2024-09-28 10:15:25 浏览: 33
在 Pandas 中,可以使用 `str.startswith()` 方法来判断字符串是否以特定的字符或字符串开头。对于判断字符串是否以 `~$` 开头,可以使用以下代码:
```python
import pandas as pd
# 假设有一个包含字符串的 DataFrame 列
df = pd.DataFrame({'col': ['~$string1', 'another string', '~$string3']})
# 使用 str.startswith() 方法判断列中每个字符串是否以 ~$ 开头
df['is_startswith_~$'] = df['col'].str.startswith('~$')
# 打印结果
print(df)
```
这段代码中,`str.startswith()` 方法用于判断每个字符串是否以 `~$` 开头。结果将被存储在名为 `is_startswith_~$` 的新列中。最后,使用 `print(df)` 可以打印出包含判断结果的 DataFrame。
注意:如果要在 Pandas 中使用正则表达式进行字符串匹配,可以使用 `str.match()` 方法。然而,`str.startswith()` 方法不直接支持正则表达式,但可以通过传递一个字符串作为参数来实现类似的功能。例如,`str.startswith('~$', regex=True)` 可以用于判断字符串是否以 `~$` 开头。
相关问题
pandas筛选特定开头的
您好!对于筛选以特定开头的值,您可以使用`str.startswith()`方法来处理。这个方法可以在pandas的Series或DataFrame中检查每个元素是否以特定的字符串开头。下面是一个示例代码:
```python
import pandas as pd
# 创建一个示例数据
data = {'Name': ['Amy', 'Bob', 'Cathy', 'David'],
'Age': [25, 30, 35, 40]}
df = pd.DataFrame(data)
# 使用str.startswith()进行筛选
filtered_df = df[df['Name'].str.startswith('A')]
# 打印筛选结果
print(filtered_df)
```
运行以上代码后,将会输出以'A'开头的姓名的行:
```
Name Age
0 Amy 25
```
希望这个例子对您有所帮助!如果您有任何其他问题,请随时提问。
pandas 取开头为
在pandas中,要取开头为某个值的数据可以使用.str.startswith()方法来进行操作。这个方法可以用于Series或DataFrame中的字符串列,用来筛选出开头为特定值的数据。
例如,我们有一个包含员工姓名的DataFrame,想要筛选出所有名字以“王”开头的员工。可以使用如下代码来实现:
```python
import pandas as pd
data = {'员工姓名': ['王小明', '张三', '王大锤', '李四', '王花花']}
df = pd.DataFrame(data)
result = df[df['员工姓名'].str.startswith('王')]
print(result)
```
以上代码将会输出如下结果:
```
员工姓名
0 王小明
2 王大锤
4 王花花
```
可以看到,通过使用.str.startswith()方法,我们成功筛选出了员工姓名开头为“王”的所有数据。
总结来说,pandas中的.str.startswith()方法非常方便,能够快速帮助我们筛选出开头为特定值的数据,是数据分析和处理中常用的方法之一。
阅读全文
相关推荐
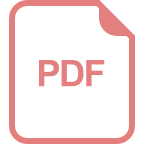
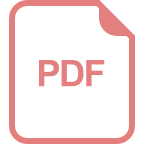
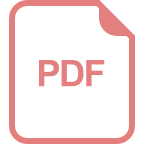
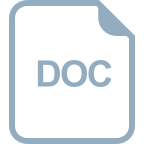

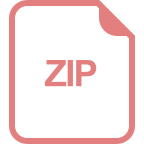










