消费者生产者进程同步Java代码
时间: 2024-06-17 17:04:16 浏览: 108
消费者生产者问题是一个经典的并发编程问题,其中生产者向共享缓冲区中插入数据,而消费者从共享缓冲区中取出数据。Java中可以使用多种方式来实现消费者生产者问题的进程同步,以下是一种常见的实现方式:
```
import java.util.LinkedList;
public class ProducerConsumer {
private LinkedList<Integer> buffer = new LinkedList<>();
private int maxSize = 10;
public void produce() throws InterruptedException {
int value = 0;
while (true) {
synchronized (this) {
while (buffer.size() == maxSize) {
wait();
}
buffer.add(value);
System.out.println("Produced " + value);
value++;
notify();
Thread.sleep(1000);
}
}
}
public void consume() throws InterruptedException {
while (true) {
synchronized (this) {
while (buffer.size() == 0) {
wait();
}
int value = buffer.removeFirst();
System.out.println("Consumed " + value);
notify();
Thread.sleep(1000);
}
}
}
}
```
在上述代码中,我们使用了一个共享缓冲区来存储生产者生成的数据。当缓冲区满时,生产者会等待,而当缓冲区为空时,消费者会等待。通过使用synchronized和wait/notify方法,我们可以确保线程安全并实现进程同步。
阅读全文
相关推荐
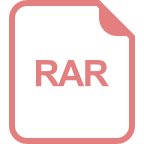
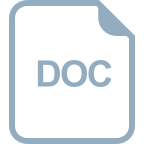













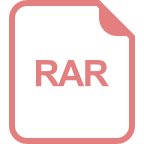