用C语言编写一个程序输入教师授课教学信息,包括教师号、姓名、性别、职称、任教课程、班级、班级数目、理论课时、实验课时。
时间: 2024-06-24 15:02:17 浏览: 6
在C语言中,你可以使用结构体和动态内存分配来创建一个程序来输入教师的教学信息。首先,定义一个结构体来存储这些属性:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义教师信息结构体
typedef struct {
int teacher_id;
char name[100];
char gender[10];
char title[100]; // 职称
char course[100]; // 任教课程
char class[100]; // 班级
int classes; // 班级数目
float theory_hours; // 理论课时
float experiment_hours; // 实验课时
} TeacherInfo;
// 函数原型
TeacherInfo* input_teacher_info();
int main() {
TeacherInfo* teacher = input_teacher_info();
// 程序接着处理输入的信息...
free(teacher); // 使用完后释放内存
return 0;
}
// 输入函数,获取教师信息并分配内存
TeacherInfo* input_teacher_info() {
TeacherInfo* new_teacher = (TeacherInfo*)malloc(sizeof(TeacherInfo));
if (!new_teacher) {
printf("Memory allocation failed.\n");
return NULL;
}
printf("请输入教师号:");
scanf("%d", &new_teacher->teacher_id);
printf("请输入姓名:");
fgets(new_teacher->name, sizeof(new_teacher->name), stdin);
// 去除末尾换行符
new_teacher->name[strcspn(new_teacher->name, "\n")] = '\0';
printf("请输入性别:");
scanf("%s", new_teacher->gender);
printf("请输入职称:");
fgets(new_teacher->title, sizeof(new_teacher->title), stdin);
new_teacher->title[strcspn(new_teacher->title, "\n")] = '\0';
printf("请输入任教课程:");
fgets(new_teacher->course, sizeof(new_teacher->course), stdin);
new_teacher->course[strcspn(new_teacher->course, "\n")] = '\0';
printf("请输入班级:");
fgets(new_teacher->class, sizeof(new_teacher->class), stdin);
new_teacher->class[strcspn(new_teacher->class, "\n")] = '\0';
printf("请输入班级数目:");
scanf("%d", &new_teacher->classes);
printf("请输入理论课时:");
scanf("%f", &new_teacher->theory_hours);
printf("请输入实验课时:");
scanf("%f", &new_teacher->experiment_hours);
return new_teacher;
}
```
相关推荐
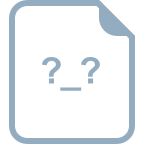














