sizeof(DWORD64)头文件
时间: 2024-09-04 07:00:19 浏览: 97
在Windows平台上,`sizeof(DWORD64)`通常不需要特定的头文件,因为`DWORD64`是Windows SDK定义的一种数据类型,它代表64位无符号长整型。`#include <windef.h>`或者`<windows.h>`这样的标准头文件可能会包含这个类型,但是实际上你直接使用`sizeof`运算符就可以获取其大小,而无需引用头文件。
然而,如果你想明确地指定一个64位整数并避免编译器警告,可以在`__int64`前加上`DWORD64`关键字,例如`sizeof(DWORD64 __int64)`。尽管如此,这并不是必需的,因为它在Windows编译器中默认就知道`__int64`是一个64位类型。
相关问题
提供一个包含常用功能的c++串口类的头文件和cpp
在C++中,编写一个包含常用功能的串口通信类可以分为两个部分:头文件(.h)和实现文件(.cpp)。这里给出一个简化的示例,假设我们叫这个类`SerialPort`,它包括基本的打开、关闭、读取和写入功能。请注意,这只是一个基础的框架,并未处理错误处理和其他复杂情况,实际应用中需要添加更多的细节和异常处理。
**serialport.h (头文件)**
```cpp
#pragma once
#include <windows.h> // 对于Windows平台下的COM库
#include <string>
class SerialPort {
private:
HANDLE m_hComPort; // 串口句柄
std::string m_portName; // 串口号
public:
// 构造函数
SerialPort(const std::string& portName);
~SerialPort(); // 析构函数,关闭串口
// 打开串口
bool open();
// 关闭串口
bool close();
// 发送数据
size_t write(const std::string& data);
// 读取数据
std::string read(size_t maxLength = 0); // 如果maxLength为0,则阻塞直到有数据可用
};
```
**serialport.cpp (实现文件)**
```cpp
#include "serialport.h"
// 构造函数
SerialPort::SerialPort(const std::string& portName) : m_portName(portName) {}
// 析构函数
SerialPort::~SerialPort() {
if (m_hComPort != INVALID_HANDLE_VALUE) {
CloseHandle(m_hComPort);
m_hComPort = INVALID_HANDLE_VALUE;
}
}
// 打开串口
bool SerialPort::open() {
m_hComPort = CreateFile(
m_portName.c_str(), // COM设备路径
GENERIC_READ | GENERIC_WRITE, // 文件属性
0, // 文件共享模式
NULL, // 安全选项
OPEN_EXISTING, // 操作模式
FILE_ATTRIBUTE_NORMAL, // 特征标志
NULL // 创建新文件
);
if (m_hComPort == INVALID_HANDLE_VALUE) {
return false;
}
return true;
}
// 关闭串口
bool SerialPort::close() {
return (CloseHandle(m_hComPort) != 0);
}
// 写入数据
size_t SerialPort::write(const std::string& data) {
DWORD bytesWritten = 0;
return WriteFile(m_hComPort, data.c_str(), data.length(), &bytesWritten, nullptr) && bytesWritten > 0;
}
// 读取数据
std::string SerialPort::read(size_t maxLength) {
char buffer[1024];
DWORD bytesRead = 0;
if (maxLength > 0) {
ReadFile(m_hComPort, buffer, maxLength, &bytesRead, nullptr);
} else {
do {
ReadFile(m_hComPort, buffer, sizeof(buffer), &bytesRead, nullptr);
} while (bytesRead > 0);
}
return std::string(buffer, bytesRead);
}
```
VC DWORD转字符串
要将 DWORD 类型的值转换为字符串,您可以使用 sprintf_s 函数或者 std::to_string 函数。
方法一:使用 sprintf_s 函数
```c
#include <windows.h>
#include <stdio.h>
DWORD dwValue = 1234; // 替换为您的 DWORD 值
char szBuffer[20]; // 缓冲区大小适当调整
sprintf_s(szBuffer, sizeof(szBuffer), "%lu", dwValue);
// 现在,szBuffer 中存储了 DWORD 值的字符串表示
```
方法二:使用 std::to_string 函数
```c
#include <windows.h>
#include <string>
DWORD dwValue = 1234; // 替换为您的 DWORD 值
std::string strValue = std::to_string(dwValue);
// 现在,strValue 中存储了 DWORD 值的字符串表示
```
请注意,这些示例假设您已经包含了相应的头文件。另外,您需要根据您的需求适当调整缓冲区大小。
希望这可以帮助到您!如果您有任何其他问题,请随时提问。
阅读全文
相关推荐
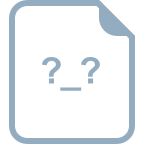
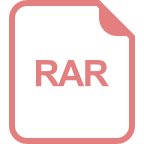
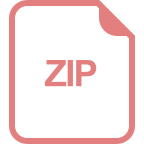
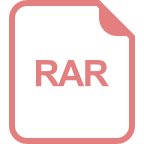
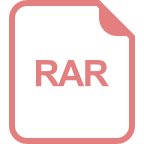
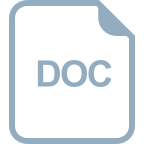
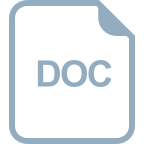






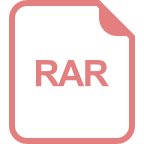
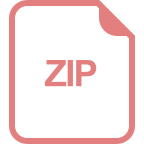