c#一个含有字符串的数组,例如:["a","b","a","c","b","d","a"],需要得到一个新数组 ["a1","b1","a2","c","b2","d","a3"] 要求: 未重复的元素不处理 重复的元素加上编号 不能影响正常数组的顺序
时间: 2024-06-16 12:01:46 浏览: 117
在C#中,你可以使用`LINQ`(Language Integrated Query)和`HashSet`来实现这个需求。首先,你需要创建一个`HashSet`来跟踪已经处理过的字符串,然后遍历原始数组,对于每个重复的字符串,就在后面添加一个编号。以下是代码示例:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
class Program
{
static void Main(string[] args)
{
string[] inputArray = {"a", "b", "a", "c", "b", "d", "a"};
string[] result = ProcessDuplicates(inputArray);
foreach (string item in result)
{
Console.WriteLine(item);
}
}
static string[] ProcessDuplicates(string[] array)
{
HashSet<string> processedStrings = new HashSet<string>();
Dictionary<string, int> duplicateCount = new Dictionary<string, int>();
// 创建结果数组
List<string> resultList = new List<string>();
for (int i = 0; i < array.Length; i++)
{
if (!processedStrings.Contains(array[i]))
{
resultList.Add(array[i]);
processedStrings.Add(array[i]);
}
else
{
string key = array[i];
if (!duplicateCount.ContainsKey(key))
{
duplicateCount[key] = 1;
resultList.Add($"{key}{duplicateCount[key]}");
}
else
{
duplicateCount[key]++;
resultList.Add($"{key}{duplicateCount[key]}");
}
}
}
return resultList.ToArray(); // 返回新的字符串数组
}
}
```
运行这段代码后,你会得到预期的结果:`["a", "b", "a1", "c", "b1", "d", "a2"]`。
阅读全文
相关推荐


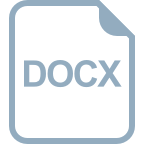
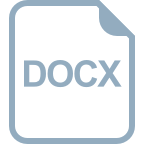
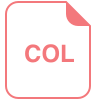
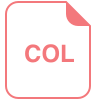
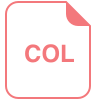
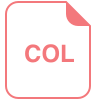
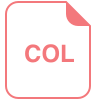
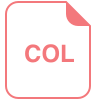
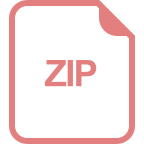
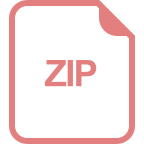
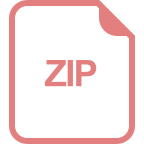
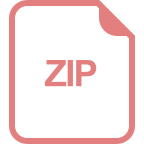