定义两个接口Eatable和Performable; 、定义一个Animal类,Tiger,Lion, Elaphant继承动物类; 、 每个动物子类都实现Eatable和Performable两个接口; 定义Zookeeper类,实现饲养员的喂养和训练功能
时间: 2024-11-05 19:25:55 浏览: 28
在面向对象编程中,可以按照以下步骤定义接口和类:
首先,我们定义两个接口 `Eatable` 和 `Performable`,它们表示动物的基本特性:
```java
// 定义Eatable接口,表示动物能吃东西
public interface Eatable {
void eat();
}
// 定义Performable接口,表示动物有表演能力
public interface Performable {
void perform();
}
```
接着,我们创建一个 `Animal` 类作为所有动物的基类,并让 `Tiger`, `Lion`, 和 `Elephant` 继承自它,同时这两个接口:
```java
// Animal类
public class Animal {
public abstract void eat(); // 约束子类实现eat方法
public abstract void perform(); // 约束子类实现perform方法
}
```
然后,`Tiger`, `Lion`, 和 `Elephant` 子类需要实现这两个接口并重写 `eat()` 和 `perform()` 方法:
```java
// Tiger类,继承Animal并实现Eatable和Performable接口
public class Tiger extends Animal implements Eatable, Performable {
@Override
public void eat() {
System.out.println("Tiger is eating");
}
@Override
public void perform() {
System.out.println("Tiger is performing its hunt");
}
}
// Lion类同理
public class Lion extends Animal implements Eatable, Performable {
// 实现eat和perform方法...
}
// Elephant类同理
public class Elephant extends Animal implements Eatable, Performable {
// 实现eat和perform方法...
}
```
最后,我们可以定义一个 `Zookeeper` 类,它实现了一个 `feed()` 和 `train()` 方法,这些方法用于照顾和训练这些动物:
```java
// Zookeeper类
public class Zookeeper {
public void feed(Animal animal) {
animal.eat();
}
public void train(Animal animal) {
animal.perform();
}
}
```
这样,Zookeeper 就可以根据接收到的不同类型的 Animal 对象(如 Tiger, Lion 或者 Elephant),分别调用其 `eat()` 和 `perform()` 方法。
阅读全文
相关推荐
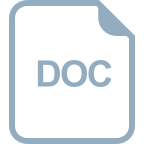
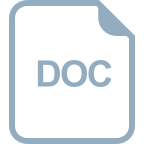
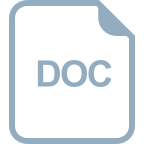
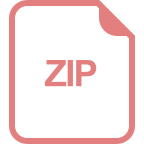
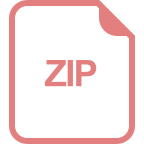
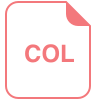









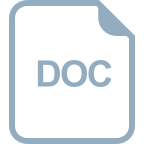
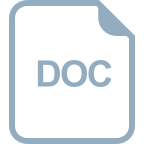
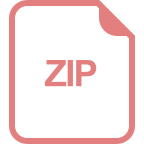
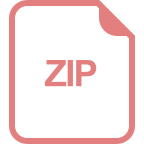