用Python代码实现MOEA/D
时间: 2024-06-01 22:06:14 浏览: 21
MOEA/D (Multi-Objective Evolutionary Algorithm Based on Decomposition)是一种多目标优化算法。它将多目标优化问题转化为多个单目标优化问题,并通过分解技术来解决这些单目标优化问题。下面是用Python实现MOEA/D的简单步骤:
1. 定义目标函数和变量范围。
2. 初始化种群,并为每个个体分配权重向量。
3. 计算每个个体的适应度值,并根据适应度值对种群进行排序。
4. 选择父代,并根据父代生成子代。
5. 对生成的子代进行修剪,保留最优的子代。
6. 更新参考点和权重向量,并将新的参考点和权重向量用于下一代进化。
下面是一个简单的Python代码示例:
```python
import numpy as np
# 定义目标函数
def obj_func(x):
f1 = x**2 + x**2
f2 = (x-1)**2 + x**2
return [f1, f2]
# 定义变量范围
x_min = np.array([-5, -5])
x_max = np.array([5, 5])
# 初始化种群和权重向量
pop_size = 100
num_obj = 2
pop = np.random.uniform(low=x_min, high=x_max, size=(pop_size, len(x_min)))
weights = np.random.uniform(low=0, high=1, size=(pop_size, num_obj))
weights = weights / np.sum(weights, axis=1)[:, None]
# 进行进化迭代
num_generations = 100
for gen in range(num_generations):
# 计算适应度值并排序
fitness = np.array([obj_func(x) for x in pop])
ranks = np.argsort(np.argsort(-fitness.dot(weights.T), axis=0), axis=0)
# 选择父代并生成子代
parents = np.random.choice(pop_size, size=pop_size)
children = np.zeros_like(pop)
for i in range(pop_size):
parent1 = parents[i]
parent2 = parents[(i+1) % pop_size]
child = pop[parent1] + 0.5*(pop[parent2]-pop[parent1])
child += 0.01*np.random.normal(size=len(x_min))
child = np.clip(child, x_min, x_max)
children[i] = child
# 修剪子代并更新参考点和权重向量
fitness_children = np.array([obj_func(x) for x in children])
ranks_children = np.argsort(np.argsort(-fitness_children.dot(weights.T), axis=0), axis=0)
pop_new = np.zeros_like(pop)
for i in range(pop_size):
if ranks_children[i] <= ranks[i]:
pop_new[i] = children[i]
else:
pop_new[i] = pop[i]
pop = pop_new
weights = np.clip(weights + 0.01*np.random.normal(size=(pop_size, num_obj)), 0, 1)
weights = weights / np.sum(weights, axis=1)[:, None]
```
相关推荐
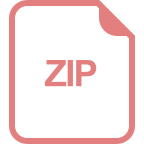
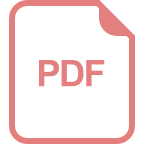














