图像分割unet pytorch
时间: 2024-01-17 10:02:02 浏览: 126
图像分割是计算机视觉领域中的重要任务,其目标是将图像分割成多个不同的区域,以便更好地理解图像中的内容。UNet是一种常用于图像分割的深度学习模型,通过使用PyTorch框架,可以更加高效地实现UNet模型。
UNet模型由编码器和解码器组成,编码器用于提取图像的特征,而解码器则用于将提取到的特征映射回原始图像尺寸。在PyTorch中,可以使用nn.Module来定义UNet模型的结构,通过继承nn.Module类来自定义编码器和解码器的网络结构,然后使用torch.nn.functional中的函数来定义模型的前向传播过程,实现特征提取和特征映射。
在训练UNet模型时,可以使用PyTorch提供的优化器和损失函数来对模型进行优化,如Adam优化器和交叉熵损失函数。通过对训练数据进行迭代训练,可以不断优化UNet模型,以使其能够更好地实现图像分割任务。
在应用UNet模型进行图像分割时,可以使用PyTorch提供的图像处理库对输入图像进行预处理,并使用训练好的UNet模型对图像进行分割,得到分割后的图像结果。这样可以快速、高效地实现图像分割任务,并且在PyTorch框架中还可以使用GPU加速来提高计算速度。
总之,通过使用PyTorch框架实现UNet模型进行图像分割任务,可以更加方便地定义模型结构、训练模型和应用模型,从而实现高效、准确的图像分割。
相关问题
unet图像分割pytorch
以下是使用PyTorch实现的UNET图像分割的示例代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class DoubleConv(nn.Module):
'''(convolution => [BN] => ReLU) * 2'''
def __init__(self, in_channels, out_channels, mid_channels=None):
super().__init__()
if not mid_channels:
mid_channels = out_channels
self.double_conv = nn.Sequential(
nn.Conv2d(in_channels, mid_channels, kernel_size=3, padding=1),
nn.BatchNorm2d(mid_channels),
nn.ReLU(inplace=True),
nn.Conv2d(mid_channels, out_channels, kernel_size=3, padding=1),
nn.BatchNorm2d(out_channels),
nn.ReLU(inplace=True)
)
def forward(self, x):
return self.double_conv(x)
class Down(nn.Module):
'''Downscaling with maxpool then double conv'''
def __init__(self, in_channels, out_channels):
super().__init__()
self.maxpool_conv = nn.Sequential(
nn.MaxPool2d(2),
DoubleConv(in_channels, out_channels)
)
def forward(self, x):
return self.maxpool_conv(x)
class Up(nn.Module):
'''Upscaling then double conv'''
def __init__(self, in_channels, out_channels, bilinear=True):
super().__init__()
# if using bilinear interpolation, the output size is half the input size
if bilinear:
self.up = nn.Upsample(scale_factor=2, mode='bilinear', align_corners=True)
self.conv = DoubleConv(in_channels, out_channels, in_channels // 2)
else:
self.up = nn.ConvTranspose2d(in_channels // 2, in_channels // 2, kernel_size=2, stride=2)
self.conv = DoubleConv(in_channels, out_channels)
def forward(self, x1, x2):
x1 = self.up(x1)
diffY = x2.size()[2] - x1.size()[2]
diffX = x2.size()[3] - x1.size()[3]
x1 = F.pad(x1, [diffX // 2, diffX - diffX // 2,
diffY // 2, diffY - diffY // 2])
x = torch.cat([x2, x1], dim=1)
return self.conv(x)
class OutConv(nn.Module):
def __init__(self, in_channels, out_channels):
super(OutConv, self).__init__()
self.conv = nn.Conv2d(in_channels, out_channels, kernel_size=1)
def forward(self, x):
return self.conv(x)
class UNet(nn.Module):
def __init__(self, n_channels, n_classes, bilinear=True):
super(UNet, self).__init__()
self.n_channels = n_channels
self.n_classes = n_classes
self.bilinear = bilinear
self.inc = DoubleConv(n_channels, 64)
self.down1 = Down(64, 128)
self.down2 = Down(128, 256)
self.down3 = Down(256, 512)
self.down4 = Down(512, 512)
self.up1 = Up(1024, 256, bilinear)
self.up2 = Up(512, 128, bilinear)
self.up3 = Up(256, 64, bilinear)
self.up4 = Up(128, 64, bilinear)
self.outc = OutConv(64, n_classes)
def forward(self, x):
x1 = self.inc(x)
x2 = self.down1(x1)
x3 = self.down2(x2)
x4 = self.down3(x3)
x5 = self.down4(x4)
x = self.up1(x5, x4)
x = self.up2(x, x3)
x = self.up3(x, x2)
x = self.up4(x, x1)
logits = self.outc(x)
return logits
```
该网络包含输入层,5个下采样层,5个上采样层和输出层。每个下采样层都包含一个最大池化层和两个卷积层。每个上采样层都包含一个卷积转置层和两个卷积层。输出层包含一个卷积层。在每个下采样和上采样层之间,都有一个双重卷积层。这个网络可以处理任意大小的输入图像,并输出与输入图像大小相同的分割结果。
u-net医学图像分割代码pytorch
U-Net是一种经典的深度卷积神经网络模型,特别适合于像医学图像分割这样的任务。在PyTorch中实现U-Net涉及以下几个步骤:
1. **引入库**:首先,你需要导入必要的库,如PyTorch、torchvision、numpy等。
```python
import torch
import torch.nn as nn
import torch.optim as optim
from torchvision import datasets, transforms
```
2. **构建U-Net架构**:U-Net由一个下采样(编码器)部分和一个上采样(解码器)部分组成,它们通过一个中心的全连接层相连。你可以使用卷积层、池化层、反卷积层(上采样可以用transpose convolution替代)以及跳跃连接(skip connections)。
```python
class UNet(nn.Module):
def __init__(self, in_channels, out_channels, num_filters=64, num_blocks=5):
super(UNet, self).__init__()
# ... 编码器部分 (downsampling)
# ... 解码器部分 (upsampling)
# ... 最后的分类层
# 初始化模型参数
model = UNet(in_channels=3, out_channels=1) # 3通道的输入,单通道的输出
```
3. **训练过程**:设置损失函数(通常是交叉熵),优化器(例如Adam或SGD),并加载数据集。
```python
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
# 训练循环
for epoch in range(num_epochs):
for inputs, targets in dataloader:
outputs = model(inputs)
loss = criterion(outputs, targets)
optimizer.zero_grad()
loss.backward()
optimizer.step()
```
4. **评估和保存模型**:在验证集上评估模型性能,并定期保存模型以便于后续使用。
```python
# 在验证集上计算准确率
val_loss = evaluate_on_val(model, val_dataloader)
# 每隔几个epoch保存模型
if (epoch + 1) % save_freq == 0 or epoch == num_epochs - 1:
torch.save(model.state_dict(), 'unet_model_epoch_{}.pt'.format(epoch))
```
阅读全文
相关推荐
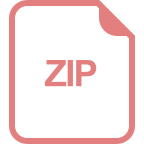
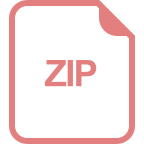
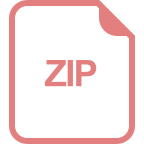
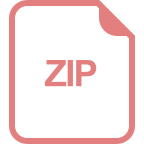
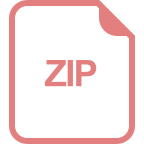
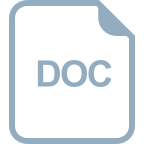
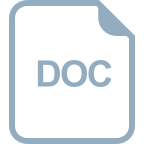
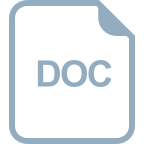




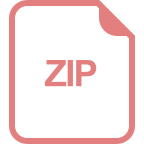
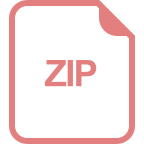
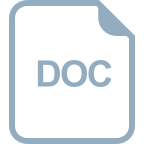

