统计灰度图像的直方图的C++实现
时间: 2024-10-06 16:05:59 浏览: 41
在C++中计算灰度图像的直方图,可以分为以下几个步骤:
1. **读取图像**: 使用OpenCV库(`opencv2`)中的函数读取图片,例如 `cv::imread()`。
```cpp
#include <opencv2/opencv.hpp>
cv::Mat image = cv::imread("path_to_your_image.jpg", cv::IMREAD_GRAYSCALE);
```
2. **创建直方图数组**: 定义一个大小为256的一维数组,代表灰度值范围从0到255,每个元素表示对应灰度值像素的数量。
```cpp
int hist[256];
```
3. **遍历像素并更新直方图**: 使用`cv::countNonZero()`或直接遍历图像矩阵来统计每个灰度级别的像素数,并更新数组。
```cpp
cv::Mat element = (cv::Mat_<uchar>(1, 256) << 0, 1, 2, ..., 254, 255); // 创建一个查找表
for (int y = 0; y < image.rows; ++y)
{
for (int x = 0; x < image.cols; ++x)
{
int gray_value = static_cast<int>(image.at<uchar>(y, x));
++hist[gray_value];
}
}
```
4. **绘制直方图**: 使用OpenCV提供的`cv::calcHist()`函数将直方图可视化,或者手动绘制。
```cpp
cv::Mat histImage;
cv::calcHist(&image, 1, nullptr, &histImage, 1, {0, 256}, cv::HIST_ARRAY);
cv::imshow("Histogram", histImage);
```
**完整示例**:
```cpp
#include <opencv2/opencv.hpp>
int main()
{
cv::Mat image = cv::imread("path_to_your_image.jpg", cv::IMREAD_GRAYSCALE);
int hist[256];
cv::Mat element = (cv::Mat_<uchar>(1, 256) << 0, 1, 2, ..., 254, 255);
for (int y = 0; y < image.rows; ++y)
{
const uchar* row = image.ptr<uchar>(y);
for (int x = 0; x < image.cols; ++x)
{
hist[static_cast<int>(*row++)]++;
}
}
// 或者使用 cv::countNonZero() 函数
// std::vector<int> histogram(image.size().width, 0);
// cv::countNonZero(image, histogram.data());
cv::Mat histImage(1, 256, CV_8UC1, cv::Scalar(0));
for (int i = 0; i < 256; ++i)
{
histImage.at<uchar>(0, i) = static_cast<uchar>(hist[i]);
}
cv::imshow("Histogram", histImage);
cv::waitKey();
return 0;
}
```
阅读全文
相关推荐
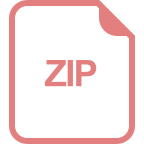
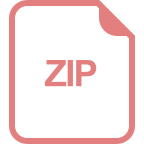
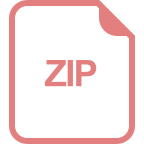
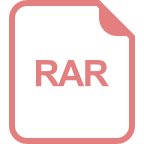
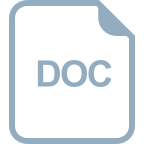
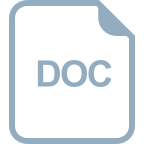
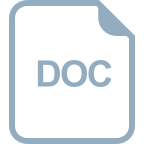
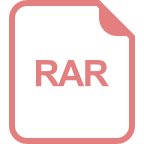
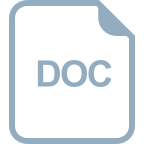
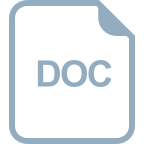
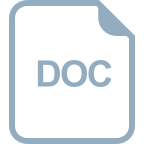
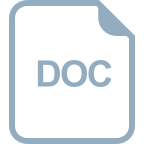
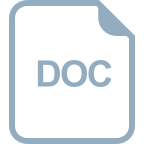
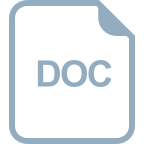
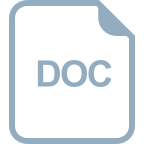



