编程计算图形的面积。程序可以用来计算圆、长方形、正方形的面积,运行时先提示用户选择图形的类型,然后对圆形要求输入半径值,对长方形要求输入长和宽的值,对正方形要求输入边长的值 计算面积并将其输出。给出C++代码
时间: 2024-09-22 19:09:53 浏览: 80
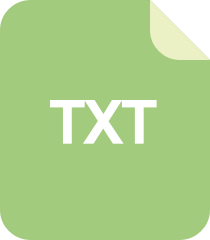
c++ 计算圆、长方形、平行四边形面积(应用‘类’‘函数重载’)
在C++中,你可以创建一个函数库来处理图形的面积计算。这里是一个简单的示例,展示了如何实现这个功能:
```cpp
#include <iostream>
#include <cmath>
// 定义一个抽象基类Shape
class Shape {
public:
virtual double getArea() const = 0; // 纯虚函数,用于声明所有形状都有一个getArea方法
};
// 具体形状类
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double getArea() const override { return M_PI * pow(radius, 2); }
};
class Rectangle : public Shape {
private:
double length, width;
public:
Rectangle(double l, double w) : length(l), width(w) {}
double getArea() const override { return length * width; }
};
class Square : public Shape {
private:
double side;
public:
Square(double s) : side(s) {}
double getArea() const override { return pow(side, 2); }
};
int main() {
std::cout << "Please choose a shape:\n";
std::cout << "1. Circle\n2. Rectangle\n3. Square\n";
int choice;
std::cin >> choice;
Shape* shape;
switch (choice) {
case 1:
double radius;
std::cout << "Enter the radius of the circle: ";
std::cin >> radius;
shape = new Circle(radius);
break;
case 2:
double length, width;
std::cout << "Enter the length and width of the rectangle: ";
std::cin >> length >> width;
shape = new Rectangle(length, width);
break;
case 3:
double side;
std::cout << "Enter the side length of the square: ";
std::cin >> side;
shape = new Square(side);
break;
default:
std::cerr << "Invalid choice!\n";
return 1;
}
double area = shape->getArea();
std::cout << "The area is: " << area << "\n";
delete shape; // 释放内存
return 0;
}
阅读全文
相关推荐
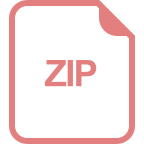
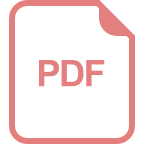








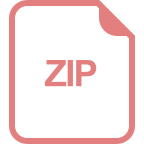
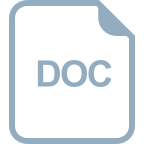





