输入一串数字,用尾接法建立链表(C语言)
时间: 2024-10-11 08:07:03 浏览: 21
尾接法(Tail Insertion)是指在创建链表时,每次新节点直接添加到现有链表的尾部。在C语言中,可以按照以下步骤实现:
1. 首先,定义一个结构体表示链表节点,包含整数值`data`和指向下一个节点的指针`next`:
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
2. 定义一个全局头结点变量`head`,初始时设为`NULL`:
```c
Node* head = NULL;
```
3. 创建链表函数,接受输入值`num`作为参数:
```c
void insertAtTail(int num) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 分配内存
if (!newNode) { // 检查内存分配是否成功
printf("Memory allocation failed.\n");
return;
}
newNode->data = num; // 设置节点值
newNode->next = NULL; // 初始化新节点指向空
// 如果链表为空或只有一个元素,直接将新节点设置为头结点
if (head == NULL || head->next == NULL) {
head = newNode;
} else {
// 否则遍历到最后一个节点并插入
Node* current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
```
4. 使用示例:
```c
int main() {
insertAtTail(1); // 插入第一个节点
insertAtTail(2);
insertAtTail(3);
// ...其他节点...
return 0;
}
```
阅读全文
相关推荐
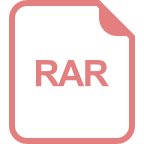
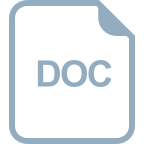
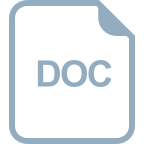















