输入一串数字,用尾接法建立链表并输出链表(C语言)
时间: 2024-10-11 22:06:53 浏览: 28
在C语言中,我们可以创建一个简单的链表结构,并使用尾接法(也称为链式附加)将输入的一串数字逐个添加到链表中。这里是一个基本的示例:
```c
#include <stdio.h>
#include <stdlib.h> // 需要头文件stdlib.h来处理内存分配
// 定义链表节点结构体
typedef struct Node {
int data; // 节点存储的数据
struct Node* next; // 指向下一个节点的指针
} Node;
// 创建新节点并将其添加到链表尾部
Node* add_to_list(Node** head, int num) {
if (*head == NULL) { // 如果链表为空
*head = (Node*)malloc(sizeof(Node)); // 分配空间给新节点
if (*head == NULL) { // 若分配失败,返回NULL
printf("Memory allocation failed.\n");
return NULL;
}
(*head)->data = num; // 设置新节点数据
(*head)->next = NULL; // 新节点无后续节点
} else {
Node* temp = *head; // 当链表非空时,从头遍历找到尾部
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = (Node*)malloc(sizeof(Node)); // 添加新节点
if (temp->next == NULL) {
printf("Memory allocation failed.\n");
return NULL;
}
temp->next->data = num; // 设置新节点数据
temp->next->next = NULL; // 新节点无后续节点
}
return *head;
}
// 打印链表
void print_list(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
int main() {
int nums[] = {1, 2, 3, 4, 5}; // 示例输入数组
Node* head = NULL;
for (int i = 0; i < sizeof(nums) / sizeof(nums[0]); i++) {
head = add_to_list(&head, nums[i]);
}
if (head != NULL) {
print_list(head);
} else {
printf("Failed to build the list.\n");
}
return 0;
}
```
在这个例子中,我们首先定义了一个链表节点结构,然后实现了`add_to_list`函数来添加元素到链表尾部。`print_list`函数用于遍历并打印整个链表。在`main`函数中,我们用输入数组nums构建链表并输出。
阅读全文
相关推荐
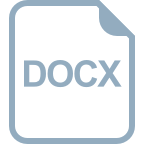
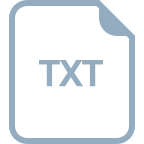
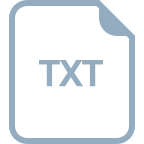















