tensorflow环境下报错AttributeError: 'Tensor' object has no attribute 'resize'
时间: 2023-11-18 21:03:31 浏览: 225
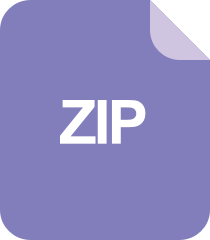
问题解决:module 'tensorflow.compat.v1' has no attribute '

在TensorFlow中,Tensor对象没有resize()方法,因此调用该方法会导致AttributeError: 'Tensor' object has no attribute 'resize'错误。如果需要更改Tensor的形状,可以使用tf.reshape()方法。例如:
```python
import tensorflow as tf
# 创建一个形状为[2, 3]的Tensor
a = tf.constant([[1, 2, 3], [4, 5, 6]])
# 使用tf.reshape()方法将其形状更改为[3, 2]
b = tf.reshape(a, [3, 2])
# 打印结果
print(b)
```
输出结果为:
```
tf.Tensor(
[[1 2]
[3 4]
[5 6]], shape=(3, 2), dtype=int32)
```
阅读全文
相关推荐
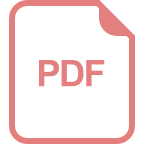
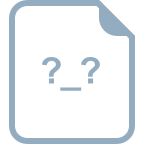











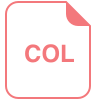




