基于stm32f4的adc采样代码
时间: 2023-09-04 13:13:01 浏览: 95
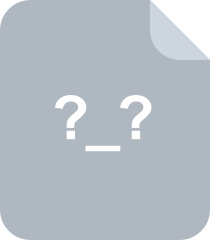
stm32四通道adc采样
以下是一个基于STM32F4的ADC采样代码的示例:
```c
#include "stm32f4xx.h"
void ADC_Init(void)
{
ADC_InitTypeDef ADC_InitStruct;
GPIO_InitTypeDef GPIO_InitStruct;
// 使能 ADC1 时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_ADC1, ENABLE);
// 使能 GPIOA 时钟
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
// 配置 ADC1 的输入引脚(PA0)
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AN;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOA, &GPIO_InitStruct);
// ADC1 初始化配置
ADC_InitStruct.ADC_Resolution = ADC_Resolution_12b;
ADC_InitStruct.ADC_ScanConvMode = DISABLE;
ADC_InitStruct.ADC_ContinuousConvMode = ENABLE;
ADC_InitStruct.ADC_ExternalTrigConvEdge = ADC_ExternalTrigConvEdge_None;
ADC_InitStruct.ADC_DataAlign = ADC_DataAlign_Right;
ADC_InitStruct.ADC_NbrOfConversion = 1;
ADC_Init(ADC1, &ADC_InitStruct);
// 配置 ADC1 的通道 0
ADC_RegularChannelConfig(ADC1, ADC_Channel_0, 1, ADC_SampleTime_15Cycles);
// 使能 ADC1
ADC_Cmd(ADC1, ENABLE);
}
uint16_t ADC_Read(void)
{
// 启动 ADC1 转换
ADC_SoftwareStartConv(ADC1);
// 等待转换完成
while(ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC) == RESET);
// 读取转换结果
return ADC_GetConversionValue(ADC1);
}
int main(void)
{
ADC_Init();
while(1)
{
uint16_t adcValue = ADC_Read();
// 对采样值进行处理
// ...
}
}
```
这段代码使用了ADC1通道0对PA0引脚进行采样,并将采样结果保存在`adcValue`变量中。你可以根据需要在while循环中对采样值进行处理。请根据自己的需求修改代码。
阅读全文
相关推荐
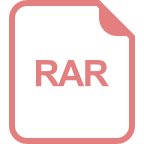
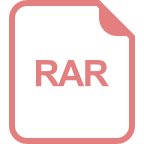



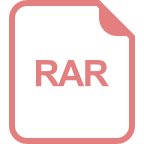
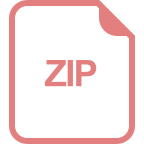
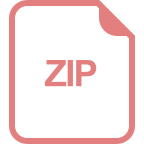
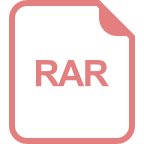
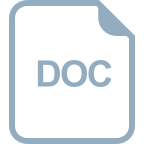
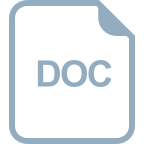




