springboot 模糊查询
时间: 2023-11-14 16:08:33 浏览: 155
可以使用 Spring Data JPA 中的 Query By Example(QBE)实现模糊查询。QBE 是一种基于实体类的动态查询方式,可以根据实体类的属性值来构建查询条件。具体实现步骤如下:
1. 创建一个实体类,该实体类中包含需要查询的属性。
2. 创建一个 Example 对象,将需要查询的属性值设置到该对象中。
3. 创建一个 ExampleMatcher 对象,设置匹配规则,如忽略大小写、模糊查询等。
4. 调用 JpaRepository 中的 findAll 方法,将 Example 对象和 ExampleMatcher 对象作为参数传入即可。
下面是一个示例代码:
```java
// 实体类
@Entity
@Table(name = "user")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private Integer age;
// getter 和 setter 略
}
// DAO 接口
public interface UserRepository extends JpaRepository<User, Long> {
}
// Service 类
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public List<User> findUsers(String name) {
User user = new User();
user.setName(name);
ExampleMatcher matcher = ExampleMatcher.matching()
.withMatcher("name", match -> match.contains().ignoreCase());
Example<User> example = Example.of(user, matcher);
return userRepository.findAll(example);
}
}
```
以上代码实现了根据用户名进行模糊查询的功能。其中,ExampleMatcher 中的 withMatcher 方法用于设置匹配规则,"name" 表示要匹配的属性名,match.contains() 表示使用模糊查询,ignoreCase() 表示忽略大小写。
阅读全文
相关推荐
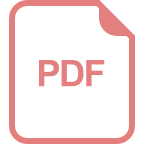
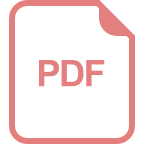
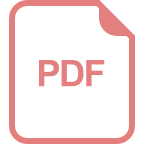















