yolov5 + opencv 车牌识别
时间: 2023-09-09 18:09:36 浏览: 123
您好!针对使用 YOLOv5 和 OpenCV 进行车牌识别的问题,我可以给出一些指导。
首先,您需要安装 YOLOv5 和 OpenCV。您可以在 GitHub 上找到 YOLOv5 的代码并进行下载。然后,使用 pip 命令安装 OpenCV。安装完成后,您可以开始使用这两个工具进行车牌识别。
下面是一个简单的步骤示例:
1. 导入所需的库:
```python
import cv2
import torch
from numpy import random
from models.experimental import attempt_load
from utils.general import non_max_suppression, scale_coords
from utils.torch_utils import select_device
```
2. 加载模型:
```python
weights = 'path/to/your/yolov5/weights'
device = select_device('')
model = attempt_load(weights, map_location=device)
stride = int(model.stride.max())
names = model.module.names if hasattr(model, 'module') else model.names
colors = [[random.randint(0, 255) for _ in range(3)] for _ in names]
```
3. 进行车牌识别:
```python
img = cv2.imread('path/to/your/image')
img = cv2.resize(img, (640, 640)) # 图像大小可以根据需求进行调整
img = torch.from_numpy(img.transpose(2, 0, 1)).float().div(255.0).unsqueeze(0)
pred = model(img)[0]
pred = non_max_suppression(pred, 0.4, 0.5)
for det in pred:
if len(det):
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], img.shape[2:]).round()
for *xyxy, conf, cls in reversed(det):
label = f'{names[int(cls)]} {conf:.2f}'
plot_one_box(xyxy, img, label=label, color=colors[int(cls)], line_thickness=3)
cv2.imshow('Result', img)
cv2.waitKey(0)
```
上述代码中,您需要将路径替换为您的 YOLOv5 权重文件和车牌图像的路径。代码会加载模型并对图像进行预测,最后将结果显示出来。
当然,这只是一个简单的示例,您可以根据自己的需求进行更多的定制和优化。希望对您有所帮助!如有任何问题,请随时提问。
阅读全文
相关推荐
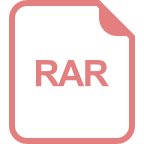
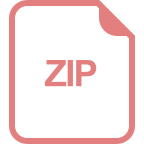
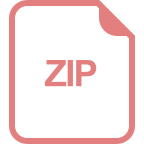
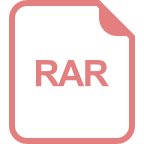
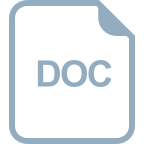
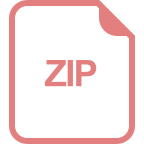
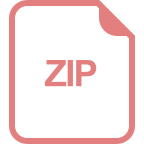
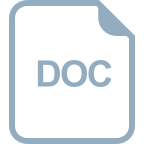

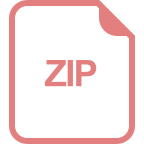
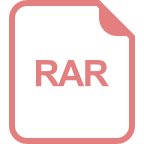
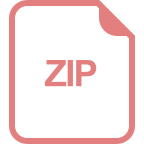
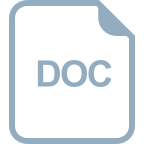
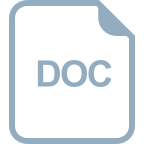



