String readLine = bufferedReader.readLine(); String[] lineArray = readLine.split(“,”);获取到的数据乱码
时间: 2024-09-20 11:10:17 浏览: 49
当你使用`readLine()`从文件输入流读取一行数据并将其存储为字符串`readLine`,然后尝试通过逗号(`", "`)分割这个字符串到数组`lineArray`时,如果原始文本存在乱码,通常是因为编码问题。这可能发生在读取过程中使用的源文件编码、目标程序的默认字符集或者两者之间不匹配。
1. 首先,确认读取文件时使用的编码:检查`BufferedReader`实例是否指定了正确的输入文件编码,如UTF-8、GBK等。例如:
```java
InputStreamReader reader = new InputStreamReader(inputStream, "UTF-8");
BufferedReader bufferedReader = new BufferedReader(reader);
```
2. 如果数据是从网络或其他非标准来源获取的,可能需要明确指定其编码格式。
3. 分割操作本身不会直接导致乱码,但如果分割后的字符串未经解码就处理,可能会显示为乱码字符。在处理每个分割得到的部分之前,应先将其转换回正确的字符集。
确保你在处理字符串之前正确地对原始数据进行了解码,比如:
```java
String decodedLine = new String(readLine.getBytes("ISO-8859-1"), "UTF-8"); // 或者你的实际编码
String[] lineArray = decodedLine.split(",");
```
相关问题
String[] s = reader.readLine().split(" ");
This line of code reads a line of input from the user using the BufferedReader object "reader" and then splits the line into an array of strings using the space character as the delimiter. The resulting array is stored in the variable "s".
For example, if the user enters the following line of text:
hello world how are you
The resulting array would be:
["hello", "world", "how", "are", "you"]
修改代码 import java.io.*; import java.util.ArrayList; import java.util.Scanner; public class jsu1 { public static void main(String[] args) throws IOException { System.out.println("输入输出图书名称:"); Scanner sc = new Scanner(System.in); String name =sc.nextLine(); File file =new File("D:/IDEA-java/src/comt/删除操作/a.txt"); FileReader FR = new FileReader(file); BufferedReader BR = new BufferedReader(FR); ArrayList<String> array =new ArrayList<>(); String line; while((line = BR.readLine()) != null){ String[] str; str=line.split(","); if(!name.equals(str[0])){ array.add(str[0]+","+str[1]+","+str[2]); array.add("\n"); } } BR.close(); FR.close(); FileWriter FW = new FileWriter(file); BufferedWriter BW = new BufferedWriter(FW); BW.write(String.valueOf(array)); BW.close(); FW.close(); } }
import java.io.*;
import java.util.ArrayList;
import java.util.Scanner;
public class jsu1 {
public static void main(String[] args) throws IOException {
System.out.println("输入要删除的图书名称:");
Scanner sc = new Scanner(System.in);
String name = sc.nextLine();
File file = new File("D:/IDEA-java/src/comt/删除操作/a.txt");
FileReader fr = new FileReader(file);
BufferedReader br = new BufferedReader(fr);
ArrayList<String> array = new ArrayList<>();
String line;
while ((line = br.readLine()) != null) {
String[] str = line.split(",");
if (!name.equals(str[0])) {
array.add(line);
}
}
br.close();
fr.close();
FileWriter fw = new FileWriter(file);
BufferedWriter bw = new BufferedWriter(fw);
for (String s : array) {
bw.write(s);
bw.newLine();
}
bw.close();
fw.close();
System.out.println("删除成功!");
}
}
修改说明:
1. 更改了输入提示信息,使其更加准确。
2. 更改了字符串拼接的方式,直接将每行的内容添加到 ArrayList 中。
3. 更改了写入文件的方式,使用 for 循环逐行写入,同时添加换行符。
4. 添加了删除成功的提示信息。
阅读全文
相关推荐
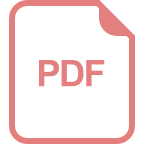
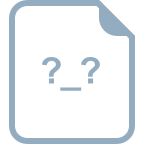
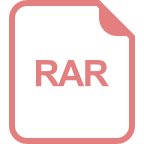



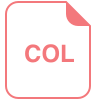
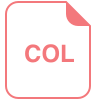
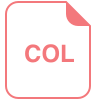
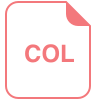
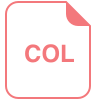






