C++ string字符替换子串
时间: 2024-12-21 12:11:18 浏览: 8
在C++中,可以使用string类的成员函数replace()来替换字符串中的子串。这个函数允许你指定要替换的子串的起始位置和长度,以及用来替换的新字符串。
下面是一个简单的例子:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
std::cout << "原始字符串: " << str << std::endl;
// 替换从位置7开始的5个字符为"Universe"
str.replace(7, 5, "Universe");
std::cout << "替换后的字符串: " << str << std::endl;
return 0;
}
```
在这个例子中,我们创建了一个初始字符串"Hello, world!",然后使用replace()函数将"world"替换为"Universe"。replace()函数的第一个参数是子串的起始位置(从0开始计数),第二个参数是要替换的字符数,第三个参数是新的子串。
输出结果将是:
```
原始字符串: Hello, world!
替换后的字符串: Hello, Universe!
```
你可以根据需要调整replace()函数的参数来替换不同位置和长度的子串。
相关问题
c++ string substring截取子串
在C++中,`std::string` 类型提供了 `substr()` 函数来截取子串。这个函数用于从给定的字符串中提取指定长度的子串。它的基本语法如下:
```cpp
std::string substr(size_type pos, size_type length = npos) const;
```
- `pos`: 从该位置开始(包含)提取子串,索引从0开始。
- `length` (可选): 提取的字符数。如果不提供,则从 `pos` 位置开始直到字符串结束。如果设置为 `npos`(是 `std::string` 类的一个特殊值),则默认截取到字符串的末尾。
例如,如果你想获取从第三个字符开始到第六个字符的子串,你可以这样使用:
```cpp
std::string str = "Hello, World!";
std::string subStr = str.substr(2, 4); // 输出: "llo,"
```
c++将string中的子串替换为另一个不一样长的子串
在 C++ 中,你可以使用 `std::string` 类的 `replace` 或 `substr` 和 `insert` 函数来替换一个子串为另一个字符串。下面是一个基本的步骤:
1. **获取原始字符串** (source_string) 和两个子串:
```cpp
std::string source_string = "Hello, World!";
std::string old_substring = "World";
std::string new_substring = "C++";
```
2. **找到旧子串的位置** (start_index) 以及它在原字符串中的长度 (length):
```cpp
size_t start_index = source_string.find(old_substring);
if (start_index == std::string::npos) {
// 如果找不到旧子串,则返回
return;
}
size_t length = old_substring.length();
```
3. **使用 `replace` 函数替换子串**:
```cpp
source_string.replace(start_index, length, new_substring);
```
4. **结果字符串就是替换后的字符串**:
```cpp
std::cout << "Replaced string: " << source_string << std::endl;
```
阅读全文
相关推荐
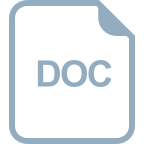
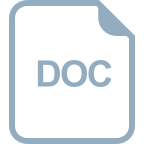
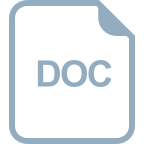
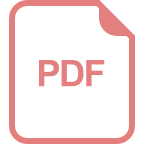
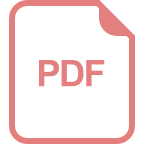
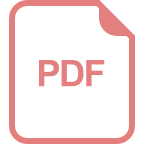
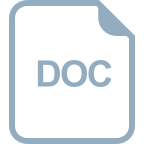









