编码:输入一段一百字的英文,统计字母的个数,及每个字母出现在的次数。构建一棵哈夫曼树,给出每个字母的哈夫曼编码,用每个字母编码对原文进行编码,将码文输出;再利用哈夫曼树对该码文进行译码,并与原文进行比较从而检验编码、译码的正确性。
时间: 2024-12-25 17:26:55 浏览: 26
在编程中,要完成这个任务,你可以按照以下步骤操作:
1. **字母计数**:
首先,你需要遍历给定的英文字符串,统计每个字符(大写字母和小写字母视为不同的字符)及其出现的次数。可以使用Python的`collections.Counter`库来轻松完成这个任务。
```python
from collections import Counter
input_string = "your_100_char_long_english_string"
char_count = Counter(input_string.lower())
```
2. **构造哈夫曼树**:
根据字符及其频率构建哈夫曼树。从最频繁的字符开始,每次合并两个最小频率的节点,直到只剩下一个节点为止。可以手动编写算法或使用现成的数据结构如`heapq`实现。
```python
import heapq
def construct_huffman_tree(freqs):
heap = [[freq, [char, '']] for char, freq in freqs.items()]
heapq.heapify(heap)
while len(heap) > 1:
left = heapq.heappop(heap)
right = heapq.heappop(heap)
combined_freq = left[0] + right[0]
combined_node = ['', left[1], right[1]]
heapq.heappush(heap, [combined_freq, combined_node])
return heap[0][1]
huffman_tree = construct_huffman_tree(char_count)
```
3. **编码和输出**:
对于每一个字符,查找其在哈夫曼树中的路径并转换为二进制编码。然后用这些编码替换原始字符串中的字符。
```python
def huffman_code(tree, char):
code = ''
current = tree
while True:
if isinstance(current, str):
return code
code += current[char]
current = current[current[char]]
encoded_string = ''.join([char+huffman_tree[char] for char in input_string])
```
4. **译码和验证**:
使用哈夫曼树的解码规则逆向处理码文,然后与原始输入字符串比较以确认编码和译码的正确性。
```python
def decode(encoded, tree):
decoded_string = ''
current = tree
for bit in encoded:
current = current[bit]
if isinstance(current, str):
decoded_string += current
break
return decoded_string
decoded_text = decode(encoded_string, huffman_tree)
print("Original text:", input_string)
print("Decoded text:", decoded_text)
```
阅读全文
相关推荐


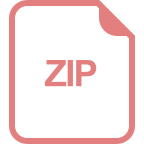












