emgu cv Image<Bgr, byte> 画圆
时间: 2024-12-08 18:24:19 浏览: 15
在Emgu CV库中,`Image<Bgr, byte>`是一个表示图像的基本数据结构,其中Bgr代表颜色模式,byte则表示像素值的范围。如果你想在这样的图像上绘制一个圆形,你可以使用`Circle`方法。以下是基本步骤:
1. 首先,你需要创建一个`Image<Bgr, byte>`实例并加载图片。
```csharp
using Emgu.CV;
using Emgu.CV.Structure;
// 加载图片
Image<Bgr, byte> image = new Image<Bgr, byte>(path_to_your_image);
```
2. 确定圆心坐标(x, y)和半径(radius)。例如:
```csharp
int centerX = your_center_x_coordinate;
int centerY = your_center_y_coordinate;
int radius = your_radius;
```
3. 使用`Circle`方法在指定位置绘制圆形。这个方法会直接修改原始图像,所以不需要额外的结果图层:
```csharp
image.Circle(new Point(centerX, centerY), radius, Bgr.Red, -1); // 参数分别为圆心、半径、颜色和线宽(-1表示填充整个圆)
```
4. 最后,如果需要保存结果,可以使用`Save`方法将处理后的图像保存到文件:
```csharp
image.Save("output_circle_image.png");
```
相关问题
emgu cv 在Image<Bgr, byte> 大图分割成小图
在Emgu CV中,将大图片分割成小图通常可以采用遍历和裁剪的方式。假设我们有一个`Image<Bgr, byte>`类型的彩色图像,我们可以按照特定的大小(例如宽度和高度的块尺寸)将其分割。以下是步骤概述:
1. **确定分割大小**:
确定你想要的小图的尺寸,比如每个小图的宽度和高度,可以用`(int)widthStep`, `(int)heightStep` 来表示。
2. **初始化变量**:
创建两个整数变量,一个用于当前行的位置(`yStart`),一个用于当前列的位置(`xStart`),并设置初始值为0。
3. **循环处理**:
使用`for`循环,直到遍历完整个大图:
a. 检查当前位置是否超出原图边界:`if (xStart + widthStep < img.Width)` 和 `if (yStart + heightStep < img.Height)`
b. 如果未超出,则创建一个新的`Image<Bgr, byte>`来保存当前小图:`var subImage = new Image<Bgr, byte>(widthStep, heightStep, img)`。
c. 裁剪原图:`img.Crop(xStart, yStart, widthStep, heightStep, subImage)`
d. 处理或保存这个子图。
e. 更新位置:`xStart += widthStep` 和 `yStart += heightStep`
4. **保存或进一步处理**:
分割后的每个子图可以根据需求保存到磁盘或继续进一步的图像处理。
```csharp
// 示例代码(简化版)
int widthStep = 100; // 横向切割间隔
int heightStep = 100; // 纵向切割间隔
for (int yStart = 0; yStart < img.Height; yStart += heightStep)
{
for (int xStart = 0; xStart < img.Width; xStart += widthStep)
{
if (xStart + widthStep <= img.Width && yStart + heightStep <= img.Height)
{
Image<Bgr, byte> subImage = img.Crop(xStart, yStart, widthStep, heightStep);
// 子图处理或保存...
}
}
}
```
记得在实际应用中处理边角部分(如果需要完整覆盖的话)以及适当添加异常处理。
c# emgucv Image<Bgr, byte> 将图像数据全部搬移到一个数组中
在C# Emgu CV库中,`Image<Bgr, byte>`是一个表示彩色BGR图像的数据结构,其中`Bgr`代表三个颜色通道(蓝色、绿色和红色),每个通道都是8位有符号整数(byte)。如果你想将这个图像的所有像素数据转移到一个数组中,你可以通过访问其像素阵列来完成。这里有一个简单的示例:
```csharp
using Emgu.CV;
using Emgu.CV.Structure;
// 假设变量image存储了Image<Bgr, byte>
Image<Bgr, byte> image = ...;
// 获取图像的宽度和高度
int width = image.Width;
int height = image.Height;
// 创建一个新的byte[]数组,大小为图像的总像素数
byte[] pixelData = new byte[width * height * 3]; // BGR图像每像素占用3个字节
// 使用LockObject来安全地访问像素,避免并发修改的问题
Mat mat = image.LockReadOnly();
Marshal.Copy(mat.ToPointer(), pixelData, 0, pixelData.Length);
image.Unlock();
// 现在pixelData数组包含了整个图像的像素数据
```
阅读全文
相关推荐
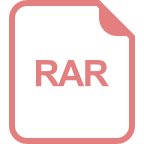
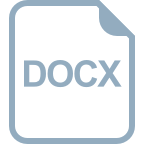
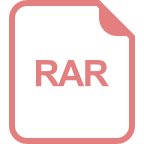













