astar算法用c++代码表示
时间: 2024-10-07 17:06:21 浏览: 33
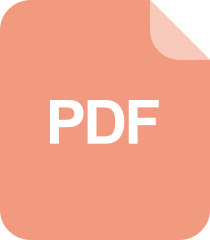
Astar算法笔记(C++代码)
A*搜索算法是一种启发式搜索算法,用于寻找从起点到终点的最短路径。以下是简单的C++代码示例,使用了优先队列`std::priority_queue`:
```cpp
#include <iostream>
#include <queue>
#include <vector>
#include <cmath>
struct Node {
int x, y;
int cost, heuristic; // cost是已走过的代价,heuristic是从当前节点到目标节点的预估代价
bool visited;
Node(int x, int y) : x(x), y(y), cost(0), heuristic(getHeuristic(x, y)), visited(false) {}
friend bool operator<(const Node& a, const Node& b) { return a.f() < b.f(); } // 比较依据f值(总代价)
};
int getHeuristic(int x, int y) {
// 这里假设曼哈顿距离作为启发函数,实际应用可能需要替换为其他适合的地图环境
return abs(x - target_x) + abs(y - target_y);
}
bool isGoal(Node node, int goal_x, int goal_y) {
return node.x == goal_x && node.y == goal_y;
}
void AStarSearch(std::vector<std::vector<int>>& grid, int start_x, int start_y, int end_x, int end_y) {
std::priority_queue<Node> openList;
openList.push(Node(start_x, start_y));
while (!openList.empty()) {
Node current = openList.top();
openList.pop();
if (isGoal(current, end_x, end_y)) {
printPath(grid, current);
return;
}
current.visited = true;
for (int dx = -1; dx <= 1; ++dx) {
for (int dy = -1; dy <= 1; ++dy) {
int nx = current.x + dx, ny = current.y + dy;
if (nx >= 0 && nx < grid.size() && ny >= 0 && ny < grid.size() && !grid[nx][ny] && !current.visited) {
openList.push(Node(nx, ny));
}
}
}
}
}
// 打印路径函数,这里仅作示意,你需要根据实际情况添加
void printPath(std::vector<std::vector<int>>& grid, Node node) {
// 实现打印路径的具体逻辑
std::cout << "Path found: ";
}
int main() {
int start_x = 0, start_y = 0, end_x = 5, end_y = 5; // 示例坐标,替换为你实际的需求
std::vector<std::vector<int>> grid; // 代表地图,你可以填充障碍物等信息
AStarSearch(grid, start_x, start_y, end_x, end_y);
return 0;
}
```
这个代码示例展示了如何在二维网格上使用A*算法进行寻路。请注意,这只是一个基本框架,实际使用时需要根据你的地图数据结构和需求进行调整。
阅读全文
相关推荐
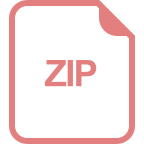
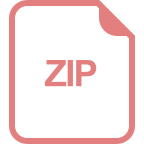

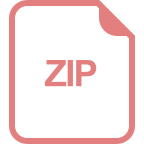
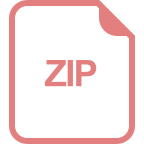
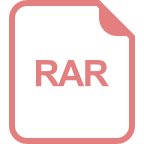
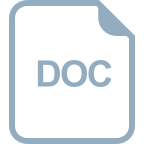
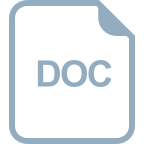


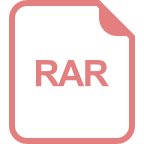
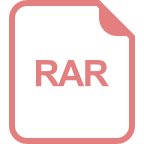
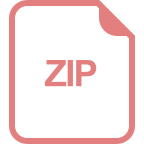



