linux 消息队列示例
时间: 2023-11-15 17:58:30 浏览: 94
Linux消息队列是一种进程间通信机制,它允许一个或多个进程向队列中添加消息,同时允许其他进程从队列中读取消息。下面是一个Linux消息队列的示例:
1. 创建消息队列:使用msgget函数创建一个新的消息队列,该函数返回一个标识符,用于后续的操作。
2. 发送消息:使用msgsnd函数向消息队列中添加消息。该函数需要指定消息队列的标识符、消息指针、消息长度和消息类型。
3. 接收消息:使用msgrcv函数从消息队列中读取消息。该函数需要指定消息队列的标识符、消息指针、消息长度、消息类型和接收标志。
下面是一个简单的Linux消息队列示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSG_SIZE 1024
struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
};
int main() {
key_t key;
int msgid;
struct msgbuf msg;
// 创建消息队列
key = ftok(".", 'a');
msgid = msgget(key, IPC_CREAT | 0666);
if (msgid == -1) {
perror("msgget");
exit(1);
}
// 发送消息
msg.mtype = 1;
strcpy(msg.mtext, "hello world!");
if (msgsnd(msgid, &msg, strlen(msg.mtext) + 1, 0) == -1) {
perror("msgsnd");
exit(1);
}
// 接收消息
if (msgrcv(msgid, &msg, MSG_SIZE, 1, 0) == -1) {
perror("msgrcv");
exit(1);
}
printf("Received message: %s\n", msg.mtext);
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(1);
}
return 0;
}
```
该示例创建了一个消息队列,向队列中添加了一条消息,然后从队列中读取了该消息,并最终删除了消息队列。
阅读全文
相关推荐
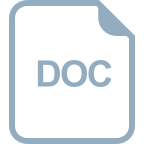
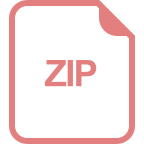
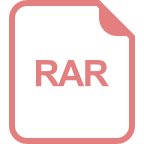
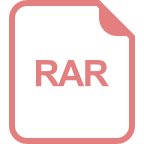
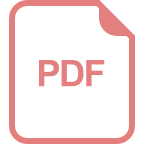
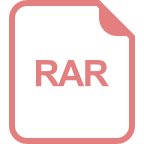
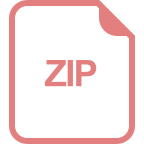
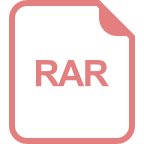
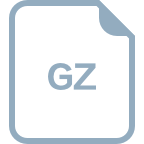
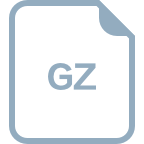
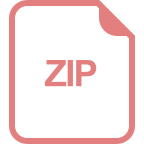
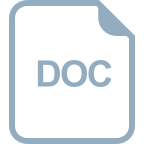
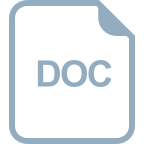


