对于任意给定一个正整数 n (大于10),输出从 1 到 n 之间,恰好只被 2 , 3 , 5 , 7 中的任意两个数字整除的数字,(如30,被2,3,5三个整数整除,则不符合要求,如21,仅被3和7整除,则符合要求)。将所有符合要求的数字从小到大输出,每个数字后跟一个空格。
时间: 2024-12-28 20:37:30 浏览: 11
要解决这个问题,我们需要遍历从1到n的所有整数,然后检查它们是否能被2、3、5或7中的任意两个数整除。我们可以通过枚举所有可能的两个因子组合来进行这个检查。这里我们可以使用一个嵌套循环来生成所有的两数对,并用模运算来判断一个数字能否被这两个因子整除。
以下是实现这个功能的C语言代码:
```c
#include <stdio.h>
int main() {
int n;
printf("Enter a positive integer greater than 10: ");
scanf("%d", &n);
if (n <= 10) {
printf("Please enter a number greater than 10.\n");
return 1; // 结束程序并提示错误
}
// 声明一个数组来存储结果
char result[100] = {0};
for (int i = 1; i <= n; ++i) {
bool isDivisibleByTwo = i % 2 == 0;
bool isDivisibleByThree = i % 3 == 0;
bool isDivisibleByFive = i % 5 == 0;
bool isDivisibleBySeven = i % 7 == 0;
// 检查至少被2, 3, 5, 或7中的任意两个整除
if ((isDivisibleByTwo && !isDivisibleByThree && !isDivisibleByFive && !isDivisibleBySeven) ||
(!isDivisibleByTwo && isDivisibleByThree && !isDivisibleByFive && !isDivisibleBySeven) ||
(!isDivisibleByTwo && !isDivisibleByThree && isDivisibleByFive && !isDivisibleBySeven) ||
(!isDivisibleByTwo && !isDivisibleByThree && !isDivisibleByFive && isDivisibleBySeven)) {
// 如果符合条件,加入结果数组
sprintf(result + strlen(result), "%d ", i);
}
}
// 移除最后一个空格
result[strlen(result) - 1] = '\0';
printf("Numbers that are divisible by exactly two of 2, 3, 5, or 7: %s\n", result);
return 0;
}
```
阅读全文
相关推荐















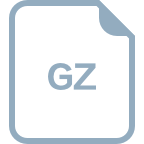