C51语言定义一个格式化输出字符串函数
时间: 2024-06-27 22:01:06 浏览: 179
C51是Microchip公司为8位AVR、Atmel AVR和Intel XMEGA等微控制器开发的C编译器,它在C语言的基础上针对嵌入式系统进行了优化。由于C51不直接支持C标准库中的所有功能,如格式化输出函数printf,所以你需要自定义一个类似的功能。
下面是一个简单的例子,展示如何定义一个格式化输出字符串的函数,这里我们称为`custom_printf`,它接受格式字符串和参数,然后使用数组来模拟printf的行为:
```c
#include <stdio.h> // 为了使用格式符类型
// 自定义的格式化输出函数
void custom_printf(const char *fmt, ...) {
va_list args;
va_start(args, fmt); // 初始化可变参数列表
// 创建一个临时数组来存储格式化后的结果
const int BUF_SIZE = 128; // 可能需要根据实际需求调整
char output[BUF_SIZE];
int i = 0;
// 使用vsprintf函数逐个处理格式符
while (*fmt != '\0') {
if (*fmt == '%') { // 当遇到%时,处理格式说明符
switch (*(fmt + 1)) { // 提取格式字符
case 'd': // 整数
i += vsprintf(output + i, "%d", va_arg(args, int));
break;
// 其他格式说明符如'e', 'f', 's', 'x' 等,自行添加处理
// ...
default:
i += snprintf(output + i, BUF_SIZE - i, "%c", *fmt); // 如果是普通字符,则直接添加
fmt++;
break;
}
fmt++; // 移动到下一个字符
} else {
i += snprintf(output + i, BUF_SIZE - i, "%c", *fmt); // 非格式说明符直接添加
fmt++;
}
}
// 最后添加换行符
i += snprintf(output + i, BUF_SIZE - i, "\n");
// 输出结果
while (i > 0) {
putchar(output[--i]);
}
va_end(args); // 结束可变参数列表
}
// 使用示例:
void main() {
custom_printf("Current time: %d:%02d:%02d\n", hour, minute, second);
}
```
阅读全文
相关推荐
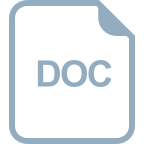
















