用c语言通过指针的方式获取数组arr里的最大的数组元素
时间: 2024-11-25 15:33:00 浏览: 4
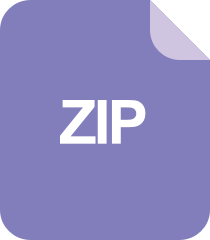
C语言——借助指针实现数组元素的逆序.zip

在C语言中,你可以使用指针来遍历数组并找到最大值。这里是一个简单的示例,展示了如何通过指针完成这个任务:
```c
#include <stdio.h>
// 定义函数,接收指向数组首元素的指针和数组长度作为参数
int findMax(int *arr, int size) {
int max = arr[0]; // 假设第一个元素为初始最大值
// 使用循环遍历数组,比较当前元素和已知的最大值
for (int i = 1; i < size; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
int main() {
int arr[] = {5, 8, 3, 9, 1}; // 假设这是你要找最大值的数组
int array_size = sizeof(arr) / sizeof(arr[0]); // 获取数组长度
int max_element = findMax(arr, array_size);
printf("Array中的最大元素是: %d\n", max_element);
return 0;
}
```
在这个例子中,`findMax`函数接受一个指向数组`arr`的指针以及数组的大小`size`。它会遍历整个数组,每次迭代都检查当前元素是否大于当前已知的最大值,如果是,则更新`max`。
阅读全文
相关推荐
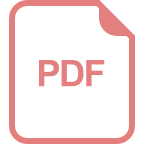
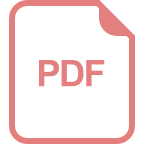
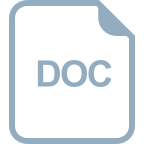











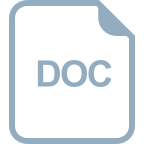


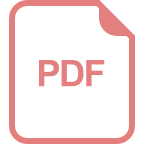
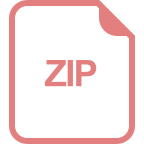