定义Mobilephone类,它是上一章节中的Telephone 的子类,除了具有 Telephone 类的属性外,它还有自己的属性如网络类型、被叫时间,同时它有自己的计算话费和显示信息的方法。最后程序中应包含一个主类来使用上述两个类并显示电话所有的信息 Java
时间: 2024-11-05 15:26:27 浏览: 21
在Java中,你可以按照以下步骤定义`Mobilephone`类,使其成为`Telephone`类的子类:
```java
// 首先,我们定义一个名为Phone的抽象类作为基础,因为我们要实现多态
public abstract class Phone {
private String phoneNumber;
// 抽象方法,需要在子类中实现
public abstract void call();
}
// 然后,定义具体的Mobilephone类,继承自Phone
public class Mobilephone extends Phone {
private String networkType; // 网络类型
private long calledTime; // 被叫时间
// 实现父类的call方法,并添加额外的行为
@Override
public void call() {
System.out.println("Calling from " + networkType);
calledTime += System.currentTimeMillis(); // 记录被叫时间
}
// 自己特有的属性和方法
public String getNetworkType() {
return networkType;
}
public long getCalledTime() {
return calledTime;
}
// 计算话费的方法,这里假设固定费用为5元每分钟,具体费用取决于实际模型
public double calculateFee(double duration) {
return 5 * duration;
}
// 显示信息的方法
public void displayInfo() {
System.out.println("Phone number: " + phoneNumber);
System.out.println("Network type: " + networkType);
System.out.println("Called time: " + calledTime + "ms");
}
}
// 主类,用于创建并使用Mobilephone对象
public class MainClass {
public static void main(String[] args) {
Mobilephone mobile = new Mobilephone();
mobile.phoneNumber = "1234567890";
mobile.networkType = "4G";
mobile.call();
mobile.displayInfo();
// 假设通话时间为10分钟
double duration = 600;
double fee = mobile.calculateFee(duration);
System.out.println("Call fee: " + fee + " yuan");
}
}
```
在这个例子中,`MainClass`展示了如何创建`Mobilephone`对象并操作其特性。通过多态,我们可以直接使用`Phone`类型的引用去处理`Mobilephone`实例,这使得代码更具灵活性。
阅读全文
相关推荐
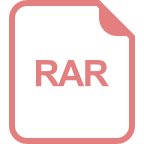
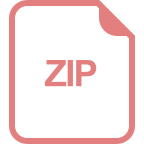
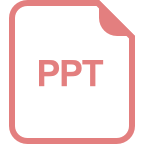
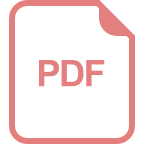



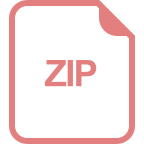
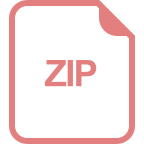
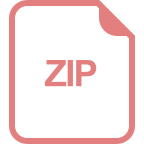