ValueError: This model has not yet been built. Build the model first by calling `build()` or by calling the model on a batch of data.
时间: 2024-02-29 22:57:04 浏览: 274
这个错误通常出现在你尝试访问一个还没有被构建的 Keras 模型的权重或属性时。在使用模型之前,必须先调用 `build()` 方法来构建模型。如果你已经调用了 `build()` 方法,但仍然遇到这个错误,可能是因为你没有传递正确的输入尺寸或数据类型。
下面是一些可能的解决方案:
1. 确保你已经成功调用了 `build()` 方法并正确地传递了输入尺寸和数据类型。可以使用 `model.summary()` 方法查看模型的结构和参数。
2. 检查你的输入数据是否与你在模型中指定的输入尺寸匹配。如果不匹配,你可以使用 `input_shape` 参数来指定正确的尺寸或调整输入数据的维度。
3. 如果你正在使用自定义层或模型,请确保它们已经被正确地实现并添加到了模型中。
4. 如果你正在使用多个 GPU 进行训练,需要确保你已经正确地设置了分布式训练参数。
如果以上方法都无法解决问题,你可以尝试重新构建模型并检查每一层的参数设置是否正确。你也可以在 Keras 社区或相关论坛上发布你的问题以获得更多帮助。
相关问题
ValueError: Exception encountered when calling layer 'model' (type Functional). Input 0 of layer "conv2d" is incompatible with the layer: expected min_ndim=4, found ndim=3. Full shape received: (None, None, None) Call arguments received by layer 'model' (type Functional): • inputs=tf.Tensor(shape=(None, None, None), dtype=float32) • training=True • mask=None显示了这个错误可以帮我改一下程序嘛
这个错误提示表明你的输入张量的维度不符合 "conv2d" 层的要求,需要将输入张量的维度调整为四维。
你可以按照下面的代码示例修改你的程序:
```python
import tensorflow as tf
# 定义你的模型
inputs = tf.keras.layers.Input(shape=(None, None, 3)) # 输入形状为 (batch_size, height, width, channels)
x = tf.keras.layers.Conv2D(32, (3, 3), activation='relu')(inputs)
x = tf.keras.layers.MaxPooling2D((2, 2))(x)
x = tf.keras.layers.Flatten()(x)
x = tf.keras.layers.Dense(64, activation='relu')(x)
outputs = tf.keras.layers.Dense(10, activation='softmax')(x)
model = tf.keras.Model(inputs=inputs, outputs=outputs)
# 编译模型
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# 训练模型
model.fit(train_dataset, epochs=10, validation_data=val_dataset)
```
在这个示例中,我们将输入张量的维度调整为四维,其中第一个维度是 batch_size,第二个和第三个维度是图片的高度和宽度,最后一个维度是图片的通道数。你可以根据你的具体情况进行调整。
ValueError: Exception encountered when calling layer "model" " f"(type Functional). Input 0 of layer "conv2d" is incompatible with the layer: expected min_ndim=4, found ndim=3. Full shape received: (None, 256, 320) Call arguments received by layer "model" " f"(type Functional): • inputs=tf.Tensor(shape=(None, 256, 320), dtype=uint8) • training=False • mask=None报错,解释
这个错误通常是因为输入数据的shape不符合模型的要求。根据报错信息,模型的第一层是`conv2d`,它要求输入数据至少是4维的。但是模型输入的数据只有3维,其shape为`(None, 256, 320)`。
解决这个问题的方法是将输入数据reshape成4维,可以在输入数据的前面增加一个维度,表示batch size,即将shape变为`(1, 256, 320, 1)`。如果是多张图片输入,则将第一个维度设置为图片数量。
代码示例:
```
import numpy as np
# 假设input_data是你的输入数据
# reshape成4维
input_data = np.expand_dims(input_data, axis=0)
input_data = np.expand_dims(input_data, axis=-1)
# 输入模型进行推理
output = model.predict(input_data)
```
阅读全文
相关推荐
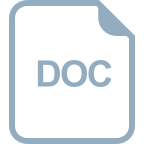
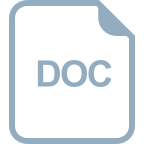
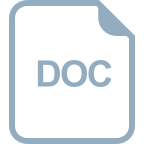
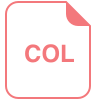
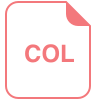







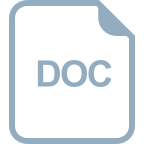
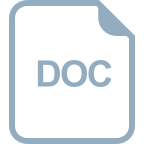
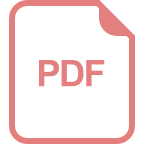
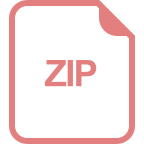