用java语言写购物系统,有超市,商品,购物者3个类,还有一个main方法。如果购物者所要购买的商品在超市中有,则提示购物者买到了商品;如果超市中没有购物者所需的商品,则提示购物者白跑了一趟,在超市中什么都没有买到。
时间: 2024-03-01 14:55:18 浏览: 26
好的,我可以为您提供一些参考代码来实现购物系统。首先是商品类:
```
public class Commodity {
private String name; // 商品名称
private double price; // 商品价格
public Commodity(String name, double price) {
this.name = name;
this.price = price;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
}
```
然后是超市类:
```
import java.util.ArrayList;
public class Supermarket {
private ArrayList<Commodity> commodities; // 超市中的商品列表
public Supermarket() {
commodities = new ArrayList<Commodity>();
}
public void addCommodity(Commodity commodity) {
commodities.add(commodity);
}
public boolean hasCommodity(String name) {
for (Commodity commodity : commodities) {
if (commodity.getName().equals(name)) {
return true;
}
}
return false;
}
}
```
最后是购物者类:
```
import java.util.Scanner;
public class Shopper {
private String name; // 购物者姓名
public Shopper(String name) {
this.name = name;
}
public void buyCommodity(Supermarket supermarket) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入您要购买的商品名称:");
String commodityName = scanner.nextLine();
if (supermarket.hasCommodity(commodityName)) {
System.out.println("恭喜您,购买成功!");
} else {
System.out.println("很遗憾,超市中没有您要购买的商品。");
}
}
}
```
在main方法中,您可以创建一个超市对象并向其中添加一些商品,然后创建一个购物者对象并让其购买商品:
```
public static void main(String[] args) {
Supermarket supermarket = new Supermarket();
supermarket.addCommodity(new Commodity("苹果", 5.0));
supermarket.addCommodity(new Commodity("香蕉", 3.0));
supermarket.addCommodity(new Commodity("西瓜", 10.0));
Shopper shopper = new Shopper("小明");
shopper.buyCommodity(supermarket);
}
```
当然,这只是一个简单的示例,您可以根据实际需求进行修改和扩展。希望能对您有所帮助!
相关推荐










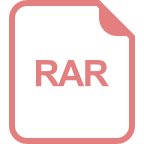
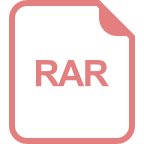