python实现sm4加解密算法
时间: 2023-09-30 12:05:06 浏览: 82
SM4是一种对称加密算法,也称为国密算法。下面是Python实现SM4加解密算法的示例代码:
```python
# coding=utf-8
import binascii
from Crypto.Cipher import AES
class SM4Cipher(object):
def __init__(self, key):
self.key = key
def encrypt(self, plaintext):
cipher = AES.new(self.key, AES.MODE_ECB)
ciphertext = cipher.encrypt(self.pad(plaintext))
return binascii.hexlify(ciphertext)
def decrypt(self, ciphertext):
cipher = AES.new(self.key, AES.MODE_ECB)
plaintext = cipher.decrypt(binascii.unhexlify(ciphertext))
return self.unpad(plaintext).decode('utf-8')
def pad(self, s):
bs = AES.block_size
return s + (bs - len(s) % bs) * chr(bs - len(s) % bs).encode()
def unpad(self, s):
return s[:-ord(s[len(s)-1:])]
if __name__ == '__main__':
key = b'0123456789abcdef'
plaintext = 'Hello, world!'
sm4 = SM4Cipher(key)
ciphertext = sm4.encrypt(plaintext.encode('utf-8'))
print('Ciphertext:', ciphertext)
decrypted_text = sm4.decrypt(ciphertext).encode('utf-8')
print('Decrypted text:', decrypted_text)
```
注意,这里使用了PyCryptodome库中的AES模块来实现SM4加解密算法。在进行加密和解密时,需要使用同一个密钥,否则无法正确解密。在加密时,需要先对明文进行填充操作,保证其长度是AES分组长度的整数倍。在解密时,需要先将填充的字节去掉,才能得到正确的明文。
相关推荐
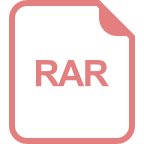
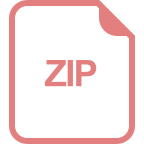














