python实现sm4加解密
时间: 2023-10-10 22:14:42 浏览: 204
Python可以使用`cryptography`库来实现SM4加解密算法。以下是一个简单的示例代码:
```python
from cryptography.hazmat.primitives import padding
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
def sm4_encrypt(key, plaintext):
# 对明文进行填充
padder = padding.PKCS7(128).padder()
padded_data = padder.update(plaintext) + padder.finalize()
# 生成随机IV
iv = os.urandom(16)
# 创建SM4加密器对象
cipher = Cipher(algorithms.SM4(key), modes.CBC(iv))
# 加密明文
encryptor = cipher.encryptor()
ciphertext = encryptor.update(padded_data) + encryptor.finalize()
return iv + ciphertext
def sm4_decrypt(key, ciphertext):
# 提取IV
iv = ciphertext[:16]
ciphertext = ciphertext[16:]
# 创建SM4解密器对象
cipher = Cipher(algorithms.SM4(key), modes.CBC(iv))
# 解密密文
decryptor = cipher.decryptor()
padded_data = decryptor.update(ciphertext) + decryptor.finalize()
# 去除填充
unpadder = padding.PKCS7(128).unpadder()
plaintext = unpadder.update(padded_data) + unpadder.finalize()
return plaintext
# 使用示例
key = b'\x01\x23\x45\x67\x89\xab\xcd\xef\xfe\xdc\xba\x98\x76\x54\x32\x10'
plaintext = b'Hello, World!'
ciphertext = sm4_encrypt(key, plaintext)
decrypted_plaintext = sm4_decrypt(key, ciphertext)
print("加密后的结果:", ciphertext)
print("解密后的结果:", decrypted_plaintext)
```
阅读全文
相关推荐
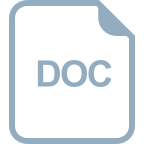
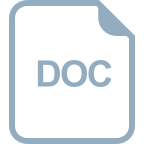
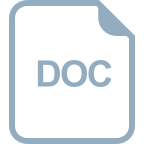








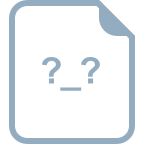
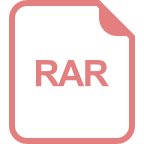




