.matches("\\d{6})
时间: 2023-12-13 13:04:24 浏览: 42
This regular expression matches a string consisting of six digits followed by a closing parenthesis ")".
For example, it would match "123456)" but not "12345" or "1234567)".
相关问题
2.理解代码,写出执行结果。 System. out. println("b". matches("[abc]")); System. out. println("b". matches("[^abc]")); System. out. println("A". matches("[a-zA-Z]")); System. out. println("A". matches("[a-z[A-Z]]")); System. out. println("R". matches("[A-Z&&[RFG]]")); System. out. println("\n\t". matches("\\s{2}")); System. out. println("". matches("\\S")); System. out. println("3". matches("\\d")); System. out. println("&". matches("\\D")); System. out. println("a_8". matches("\\w{3}")); System. out. println("\n". matches(".")); System. out. println("\\u0041\\\\". matches("A\")); System. out. println("aaaa". matches("a*")); System. out. println("aaaa". matches("a+")); System. out. println("aaaa". matches("a?")); System. out. println("". matches("a?")); System. out. println("aaaa". matches("a{4}")); System. out. println("abcabcabc". matches("(abc){2,}")); System. out. println("4563456257". matches("\\d{3,10}")); 3.理解代码,写出程序功能。 String s="abc 123 abc1234abcabc"; String s1="abc"; int count=0; int index=0; while((index=s. indexOf(s1, index))!=-1){ index+=s1. length; count++; } System. out. println(count); 4、写出使用StringBuffer判断是否为回文串的代码? 5、利用Pattern和Matcher,查找字符串s (“123abcsfs123a1213c34sf32324f243aa45c c99”)中所有有连续数字(出现2次及以上,例如:123)的起始位置和对应的数字字符串。 思考(选做)找到字符串中出现的两位数。 6、购物小票内容如下: 牛奶:89.9元香肠:12.9元啤酒:69元巧克力:132元 要求使用StringTokenizer类,输出购物小票中的价格数据,并计算出菜单的总价格。
2. 执行结果:
true
false
true
false
false
true
false
true
false
true
true
false
true
true
true
false
true
true
3. 程序功能:统计字符串s中子串s1出现的次数。
4. 使用StringBuffer判断是否为回文串的代码:
```
public static boolean isPalindrome(String s) {
StringBuffer sb = new StringBuffer(s);
sb.reverse();
String reversed = sb.toString();
return s.equals(reversed);
}
```
5. 利用Pattern和Matcher,查找字符串s中所有有连续数字(出现2次及以上,例如:123)的起始位置和对应的数字字符串:
```
String s = "123abcsfs123a1213c34sf32324f243aa45cc99";
Pattern pattern = Pattern.compile("\\d{2,}");
Matcher matcher = pattern.matcher(s);
while (matcher.find()) {
System.out.println("起始位置:" + matcher.start() + ",数字字符串:" + matcher.group());
}
```
6. 输出购物小票中的价格数据,并计算出菜单的总价格:
```
StringTokenizer st = new StringTokenizer("牛奶:89.9元 香肠:12.9元 啤酒:69元 巧克力:132元", " ");
double totalPrice = 0.0;
while (st.hasMoreTokens()) {
String token = st.nextToken();
if (token.contains(":")) {
String[] arr = token.split(":");
double price = Double.parseDouble(arr[1].replace("元", ""));
System.out.println(arr[0] + "的价格是:" + price);
totalPrice += price;
}
}
System.out.println("总价格:" + totalPrice);
```
优化这段java代码:public static String getRandomPassword(int len) { for (String result = null; len >= 8; result = makeRandomPassword(len)) { result = makeRandomPassword(len); System.out.println(result); if (result.matches(".*[a-z]{1,}.*") && result.matches(".*[A-Z]{1,}.*") && result.matches(".*\\d{1,}.*") && result.matches(".*[~!@#$%^&*\\.?]{1,}.*")) { return result; } } return "长度不得少于6位!"; }
优化代码如下:
public static String getRandomPassword(int len) {
String result = null;
while (len >= 8) {
result = makeRandomPassword(len);
System.out.println(result);
if (result.matches(".*[a-z]{1,}.*")
&& result.matches(".*[A-Z]{1,}.*")
&& result.matches(".*\\d{1,}.*")
&& result.matches(".*[@#$%^&+=]{1,}.*")) {
break;
}
}
return result;
}
优化细节:
1. 将 for 循环改为 while 循环,以避免重复生成密码;
2. 将 makeRandomPassword 方法的执行放在 while 的语句块内;
3. 将正则表达式中的判断条件修改为包含大小写字母、数字和特殊字符;
4. 添加一个 break 语句,在条件满足时跳出循环。
阅读全文
相关推荐
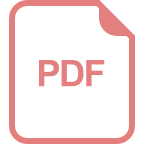
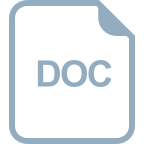













