YOUR_SPEECH_TO_TEXT_API_URL怎么找到
时间: 2023-07-14 11:59:25 浏览: 81
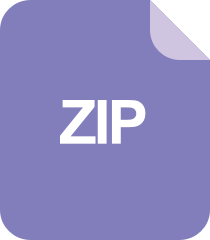
IBM_Watson_Speech_to_Text:利用IBM Watson的服务将实时输入语音转换为文本
YOUR_SPEECH_TO_TEXT_API_URL 是一个占位符,代表你自己的语音转文本 API 的 URL 地址。这个 URL 地址通常是由你使用的语音转文本服务提供的。如果你还没有使用任何语音转文本服务,你需要先选择并注册一个合适的服务提供商。
一些常见的语音转文本服务提供商包括 Google Cloud Speech-to-Text、IBM Watson Speech to Text、Microsoft Azure Speech to Text 等等。你可以前往它们的官方网站,了解他们的服务和API,并注册账号获取你自己的 API URL。
一旦你获得了自己的语音转文本 API URL,就可以将 YOUR_SPEECH_TO_TEXT_API_URL 替换为你自己的地址,以便在你的代码中使用。记得在使用 API 时,还需要提供其他必要的认证信息,如 API 密钥等。
总结来说,YOUR_SPEECH_TO_TEXT_API_URL 是一个占位符,代表你自己的语音转文本 API 的 URL 地址,具体的 URL 地址需要从你使用的语音转文本服务提供商处获得。
阅读全文
相关推荐
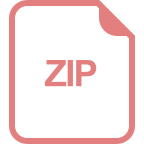
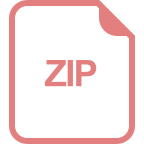
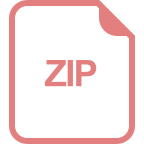
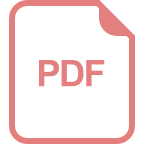
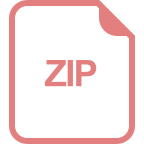











