c语言 arp报文发送程序
时间: 2023-10-05 12:05:10 浏览: 115
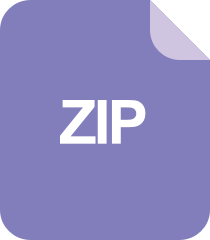
发送arp程序
下面是一个简单的 C 语言 ARP 报文发送程序示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <sys/socket.h>
#include <sys/ioctl.h>
#include <net/if.h>
#include <net/if_arp.h>
#define ARP_REQUEST 1
#define ARP_REPLY 2
struct arp_packet {
unsigned short hardware_type;
unsigned short protocol_type;
unsigned char hardware_size;
unsigned char protocol_size;
unsigned short opcode;
unsigned char sender_mac[6];
unsigned char sender_ip[4];
unsigned char target_mac[6];
unsigned char target_ip[4];
};
void get_mac_address(char *interface, unsigned char *mac) {
struct ifreq ifr;
int sock = socket(AF_INET, SOCK_DGRAM, 0);
strcpy(ifr.ifr_name, interface);
ioctl(sock, SIOCGIFHWADDR, &ifr);
memcpy(mac, ifr.ifr_hwaddr.sa_data, 6);
close(sock);
}
void get_ip_address(char *interface, unsigned char *ip) {
struct ifreq ifr;
int sock = socket(AF_INET, SOCK_DGRAM, 0);
strcpy(ifr.ifr_name, interface);
ioctl(sock, SIOCGIFADDR, &ifr);
memcpy(ip, &((struct sockaddr_in *)&ifr.ifr_addr)->sin_addr, 4);
close(sock);
}
void send_arp_request(char *interface, char *target_ip) {
int sock = socket(AF_PACKET, SOCK_RAW, htons(ETH_P_ARP));
unsigned char source_mac[6];
get_mac_address(interface, source_mac);
unsigned char source_ip[4];
get_ip_address(interface, source_ip);
unsigned char broadcast_mac[6] = {0xff, 0xff, 0xff, 0xff, 0xff, 0xff};
unsigned char target_ip_addr[4];
inet_aton(target_ip, (struct in_addr *)target_ip_addr);
struct arp_packet request;
request.hardware_type = htons(ARPHRD_ETHER);
request.protocol_type = htons(ETH_P_IP);
request.hardware_size = 6;
request.protocol_size = 4;
request.opcode = htons(ARP_REQUEST);
memcpy(request.sender_mac, source_mac, 6);
memcpy(request.sender_ip, source_ip, 4);
memset(request.target_mac, 0, 6);
memcpy(request.target_ip, target_ip_addr, 4);
struct sockaddr_ll socket_address;
socket_address.sll_family = PF_PACKET;
socket_address.sll_protocol = htons(ETH_P_ARP);
socket_address.sll_ifindex = if_nametoindex(interface);
socket_address.sll_hatype = ARPHRD_ETHER;
socket_address.sll_pkttype = PACKET_BROADCAST;
socket_address.sll_halen = ETH_ALEN;
memcpy(socket_address.sll_addr, broadcast_mac, 6);
sendto(sock, &request, sizeof(request), 0, (struct sockaddr *)&socket_address, sizeof(socket_address));
close(sock);
}
int main(int argc, char **argv) {
if (argc != 3) {
printf("Usage: %s <interface> <target_ip>\n", argv[0]);
return 1;
}
send_arp_request(argv[1], argv[2]);
printf("ARP request sent to %s\n", argv[2]);
return 0;
}
```
这个程序使用了 Linux 下的原始套接字接口和 ioctl 函数来获取本机的 MAC 地址和 IP 地址,并利用这些信息构造并发送了一个 ARP 请求报文。需要注意的是,发送 ARP 报文需要有 root 权限。
阅读全文
相关推荐

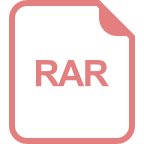
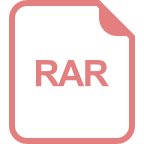
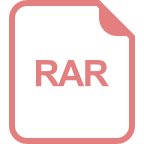
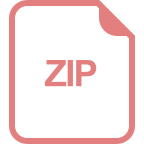
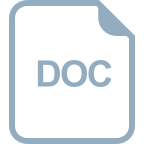
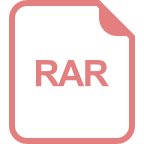
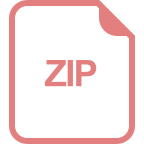
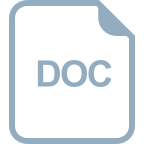
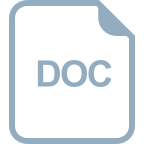
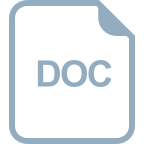
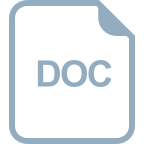
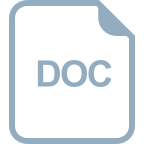

