写一个在jupyter notebooks上基于DWT图像压缩的代码,并能计算压缩率
时间: 2024-02-17 19:02:11 浏览: 149
好的,以下是基于DWT的图像压缩代码:
```python
import pywt
import numpy as np
from matplotlib import pyplot as plt
from PIL import Image
def dwt_compress(image_path, compression_ratio):
# 加载图像
image = Image.open(image_path)
image_array = np.array(image)
# 对图像进行2级DWT分解
coeffs2 = pywt.dwt2(image_array, 'haar')
LL, (LH, HL, HH) = coeffs2
LL2, (LH2, HL2, HH2) = pywt.dwt2(LL, 'haar')
# 获取DWT系数
coeffs = [LL2, LH2, HL2, HH2]
# 对DWT系数进行压缩
for i in range(len(coeffs)):
# 将系数按照重要性进行排序
sorted_coeffs = np.sort(np.abs(coeffs[i]), axis=None)[::-1]
# 计算需要保留的系数个数
num_coeffs = int(len(sorted_coeffs) * compression_ratio)
# 将剩余的系数置为0
threshold = sorted_coeffs[num_coeffs]
coeffs[i][np.abs(coeffs[i]) < threshold] = 0
# 对DWT系数进行2级反DWT重构
LLr = pywt.idwt2((LL2, (LH2, HL2, HH2)), 'haar')
image_array_reconstructed = pywt.idwt2((LLr, (LH, HL, HH)), 'haar')
# 显示原图和压缩后的图像
fig, axs = plt.subplots(1, 2, figsize=(10, 5))
axs[0].imshow(image_array, cmap='gray')
axs[0].set_title('Original Image')
axs[1].imshow(image_array_reconstructed, cmap='gray')
axs[1].set_title('Reconstructed Image')
plt.show()
# 计算压缩率
original_size = image_array.shape[0] * image_array.shape[1]
compressed_size = np.sum([coeffs[i].size for i in range(len(coeffs))])
compression_ratio = compressed_size / original_size
print('Compression ratio:', compression_ratio)
```
代码中的`dwt_compress`函数接收图像路径和压缩比作为输入,输出压缩后的图像,并计算压缩率。在函数内部,我们首先加载图像,然后进行2级DWT分解。接着,我们对每个DWT系数进行压缩,具体的方法是,将系数按照绝对值大小进行排序,然后保留前N个系数,将剩余的系数置为0。最后,我们对压缩后的系数进行2级反DWT重构,得到压缩后的图像。
下面是一个示例,演示如何对一个图像进行压缩:
```python
dwt_compress('lena.png', 0.05)
```
这将对`lena.png`图像进行压缩,保留原始图像的5%的DWT系数。运行代码后,将显示原始图像和压缩后的图像,并输出压缩率。
阅读全文
相关推荐
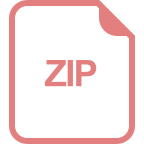









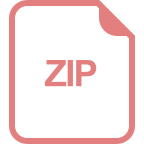
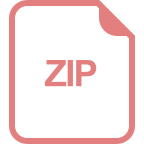
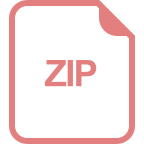