对txt文件使用stoplist.txt去除停用词,且进行中文分词精确模式处理,保存结果文件。最后提取关键词及其出现次数、频率。,并保存为xlsx文件
时间: 2024-05-12 20:19:13 浏览: 5
以下是Python代码实现:
```python
import jieba
import openpyxl
# 读取停用词表
stop_words = set()
with open('stoplist.txt', 'r', encoding='utf-8') as f:
for line in f:
stop_words.add(line.strip())
# 读取原始文本文件
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 中文分词,去除停用词
words = list(jieba.cut(text))
words = [word for word in words if word not in stop_words]
# 统计关键词出现次数
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 计算关键词频率
total_words = len(words)
for word in word_count:
word_count[word] = {'count': word_count[word], 'frequency': word_count[word] / total_words}
# 排序,提取前50个关键词
sorted_word_count = sorted(word_count.items(), key=lambda x: x[1]['count'], reverse=True)[:50]
# 保存结果到xlsx文件
wb = openpyxl.Workbook()
ws = wb.active
ws.title = 'Keywords'
ws['A1'] = '关键词'
ws['B1'] = '出现次数'
ws['C1'] = '频率'
for row, (word, count) in enumerate(sorted_word_count, start=2):
ws.cell(row=row, column=1, value=word)
ws.cell(row=row, column=2, value=count['count'])
ws.cell(row=row, column=3, value=count['frequency'])
wb.save('keywords.xlsx')
```
上述代码中,首先读取停用词表stoplist.txt,并使用set()函数将其转换为集合,方便后续快速判断一个单词是否为停用词。然后,读取原始文本文件text.txt,使用jieba库对中文文本进行分词,得到分词后的单词列表words。接着,使用列表推导式将words中的停用词去除。然后,使用字典word_count统计每个单词出现的次数,并计算单词出现的频率。最后,使用sorted()函数将word_count按照出现次数从大到小排序,并取前50个关键词。最后,将关键词、出现次数和频率保存到xlsx文件中。
相关推荐











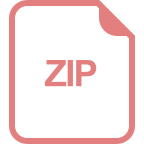
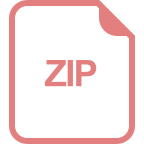
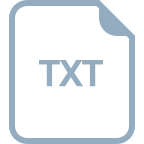