解释代码def getStopList(): # 获取停用词表,这里给出的是网易云常用的部分停用词,也可以从本地读取文件 stopList = ['不要', '个人', '这里', '有些', '完全', '头像', '搜索', '还是', '那里', '看到', '不到', '回复', '歌手', '虽然', '网易云', '怎么', '曲子', '这首', '歌单', '不过',
时间: 2023-08-20 09:42:19 浏览: 69
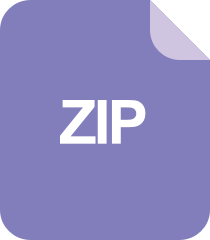
常用停用词(哈工大停用词表、百度停用词表、四川大学停用词表、中文停用词表)

这段代码定义了一个名为 `getStopList` 的函数,用于获取停用词表。在函数内部,定义了一个包含常用停用词的列表 `stopList`。这些停用词是从网易云音乐中提取的,但也可以从本地文件中读取。停用词是指在文本分析中被过滤掉的常用词汇,因为它们通常不包含有用的信息,或者会干扰文本分析的结果。
阅读全文
相关推荐
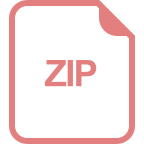
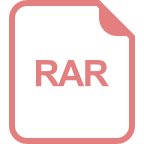















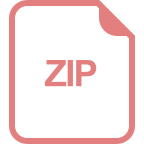