如何用c++实现打飞机的代码
时间: 2024-06-10 09:05:57 浏览: 11
这是一个经典的小游戏,可以使用 C 语言和一些库函数来实现。下面是实现打飞机游戏的基本步骤:
1. 导入必要的库文件,例如stdio.h, conio.h, graphics.h, 以及dos.h。
2. 初始化图形窗口,与用户交互,获取用户输入的操作命令。
3. 设计飞机的移动、射击和敌机的移动、死亡等动作。
4. 通过循环实现游戏的主逻辑。
下面是一份简单的 C 语言代码示例来实现打飞机游戏:
```c
#include<stdio.h>
#include<conio.h>
#include<graphics.h>
#include<dos.h>
void shoot(int x, int y)
{
moveto(x,y);
lineto(x,y-20);
}
void plane(int x, int y)
{
setcolor(YELLOW);
moveto(x,y);
lineto(x+20,y-20);
lineto(x+60,y-20);
lineto(x+80,y);
lineto(x+60,y+20);
lineto(x+20,y+20);
lineto(x,y);
shoot(x+40,y+20);
}
void enemy(int x,int y)
{
setcolor(RED);
moveto(x,y);
lineto(x+20,y+20);
lineto(x+40,y);
lineto(x+20,y-20);
lineto(x,y);
}
void main()
{
int gd=DETECT, gm;
initgraph(&gd, &gm, "");
int pl_x = 150, en_x1 = 10, en_x2 = 200;
int pl_y = 400, en_y1 = 20, en_y2 = 50;
int i, j, et=5, score = 0;
int end_game = 0;
char input;
while (end_game != 1)
{
printf("Score: %d \n", score);
printf("Use 'a' and 'd' to move the plane, press 'x' to exit.\n");
settextstyle(DEFAULT_FONT,HORIZ_DIR,2);
outtextxy(50,5,"attack of the enemys");
plane(pl_x,pl_y);
enemy(en_x1,en_y1);
enemy(en_x2,en_y2);
delay(100);
cleardevice();
if(kbhit())
{
input=getch();
if(input=='a') pl_x-=10;
if(input=='d') pl_x+=10;
if(input=='x') end_game = 1;
}
en_x1+=7;
en_x2+=6;
en_y1+=et;
en_y2+=et;
if(en_x1 > 639)
{
en_x1=10;
en_y1=20;
score += 10;
}
if(en_x2 > 639)
{
en_x2=10;
en_y2=50;
score += 10;
}
if(en_y1 >= 480 || en_y2 >= 480)
{
end_game = 1;
printf("\n Game Over \n");
printf("Your Final Score: %d \n", score);
}
if((pl_x+40) >= en_x1 && (pl_x+40) <= (en_x1+40) && en_y1 >= (pl_y-20))
{
end_game = 1;
printf("\n Game Over \n");
printf("Your Final Score: %d \n", score);
}
if((pl_x+40) >= en_x2 && (pl_x+40) <= (en_x2+40) && en_y2 >= (pl_y-20))
{
end_game = 1;
printf("\n Game Over \n");
printf("Your Final Score: %d \n", score);
}
}
getch();
closegraph();
}
```
以上代码实现的是简单的控制矩形飞机移动与敌机相互碰撞的得分游戏,欢迎根据自己的流程进行相应的代码实现。
相关推荐
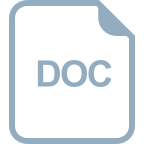
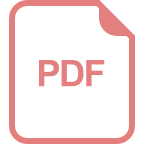










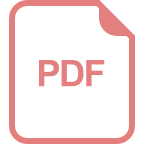
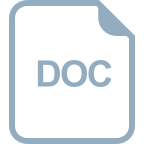
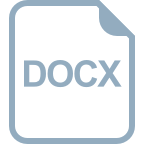
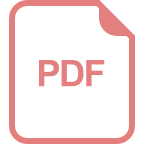