java发送websocket请求
时间: 2023-08-04 22:38:52 浏览: 136
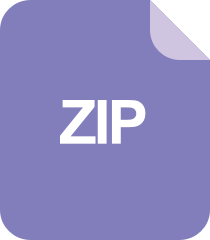
java websocket

在Java中发送WebSocket请求可以使用Java API中的Java WebSocket API。下面是一个简单的例子:
```java
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.WebSocket;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.TimeUnit;
public class WebSocketExample {
public static void main(String[] args) throws InterruptedException, ExecutionException {
HttpClient client = HttpClient.newHttpClient();
WebSocket webSocket = client.newWebSocketBuilder()
.buildAsync(URI.create("wss://example.com"), new WebSocketListener())
.get(10, TimeUnit.SECONDS);
CompletableFuture<Void> completionFuture = new CompletableFuture<>();
webSocket.sendText("Hello, world!", true).thenRun(() -> completionFuture.complete(null));
completionFuture.get();
}
static class WebSocketListener implements WebSocket.Listener {
@Override
public void onOpen(WebSocket webSocket) {
System.out.println("WebSocket opened");
}
@Override
public CompletionStage<?> onText(WebSocket webSocket, CharSequence data, boolean last) {
System.out.println("Received text: " + data);
return null;
}
@Override
public void onError(WebSocket webSocket, Throwable error) {
System.out.println("WebSocket error: " + error.getMessage());
}
@Override
public void onClose(WebSocket webSocket, int statusCode, String reason) {
System.out.println("WebSocket closed with code " + statusCode + ", reason: " + reason);
}
}
}
```
以上代码使用Java标准库中的HttpClient和WebSocket API来发送WebSocket请求。在WebSocket构建器中指定WebSocket的URI和一个WebSocket监听器,然后调用sendText方法来发送消息。在监听器中实现onText方法来处理接收到的消息。
阅读全文
相关推荐
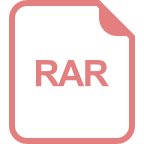








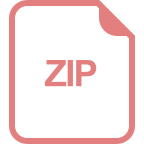


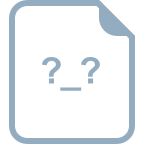
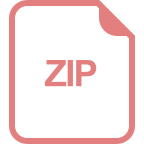
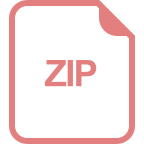