用C++写一个实现散列表(开散列)查找操作的代码
时间: 2024-02-22 09:57:47 浏览: 20
以下是使用C++实现开散列查找操作的代码:
```c++
#include <iostream>
#include <list>
using namespace std;
// 哈希表的大小
#define TABLE_SIZE 10
class HashTable {
private:
// 哈希表的桶,使用链表实现
list<pair<int, int>> table[TABLE_SIZE];
// 哈希函数,将关键字映射到桶的位置
int hash(int key) {
return key % TABLE_SIZE;
}
public:
// 插入操作
void insert(int key, int value) {
int index = hash(key);
table[index].push_back({key, value});
}
// 查找操作
int search(int key) {
int index = hash(key);
for (auto it = table[index].begin(); it != table[index].end(); it++) {
if (it->first == key) {
return it->second;
}
}
return -1;
}
};
int main() {
HashTable ht;
// 插入数据
ht.insert(1, 10);
ht.insert(2, 20);
ht.insert(3, 30);
ht.insert(11, 110);
ht.insert(12, 120);
// 查找数据
cout << ht.search(1) << endl; // 输出:10
cout << ht.search(2) << endl; // 输出:20
cout << ht.search(3) << endl; // 输出:30
cout << ht.search(11) << endl; // 输出:110
cout << ht.search(12) << endl; // 输出:120
cout << ht.search(4) << endl; // 输出:-1(不存在)
return 0;
}
```
以上代码中,使用了一个桶数组来表示哈希表,每个桶对应一个链表,用于存储哈希冲突的元素。在插入操作中,根据哈希函数将关键字映射到桶的位置,然后将元素插入到对应桶的链表中。在查找操作中,同样根据哈希函数将关键字映射到桶的位置,然后遍历对应桶的链表,查找目标元素。
相关推荐
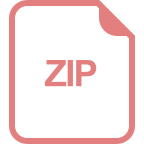
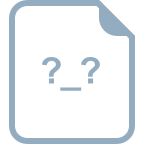
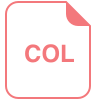
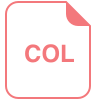
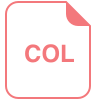
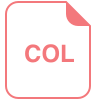
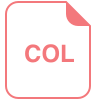









