python实现高斯噪声信号
时间: 2023-07-11 18:23:55 浏览: 103
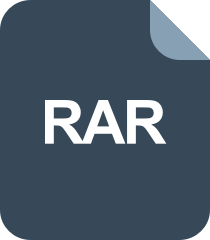
分形高斯噪声生成软件-exe
下面是 Python 实现高斯噪声信号的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 生成高斯噪声信号
def gaussian_noise(mu, sigma, length):
noise = np.random.normal(mu, sigma, length)
return noise
# 生成信号
x = np.linspace(0, 1, 100)
y = np.sin(2 * np.pi * x)
# 添加高斯噪声
mu = 0 # 均值
sigma = 0.1 # 标准差
noise = gaussian_noise(mu, sigma, len(x))
y_noise = y + noise
# 绘制信号和噪声信号
plt.plot(x, y, label='Original signal')
plt.plot(x, y_noise, label='Signal with Gaussian noise')
plt.legend()
plt.show()
```
这段代码中,`gaussian_noise` 函数用于生成高斯噪声信号,其中 `mu` 是噪声的均值,`sigma` 是噪声的标准差,`length` 是噪声信号的长度。`x` 和 `y` 是原始信号,`y_noise` 是添加了高斯噪声的信号。最后使用 `matplotlib` 库绘制了原始信号和噪声信号的图像。
阅读全文
相关推荐
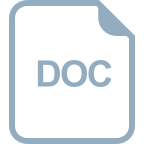
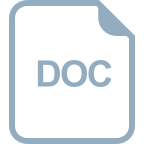















