帮我用c语言写一段代码,要求如下:函数名为void * malloc_safe;形式参数为int size; Like malloc, allocate space of size bytes on the heap. The differences are that a. anything is wrong, quit the program; b. param is int, unlike the unsigned int parameter of malloc. It is a sensitive and alerting version of malloc. ;If size is 0 or negative, some error message is printed and exit the program. If anything wrong ( malloc returns NULL), print some error message and exit the whole program. When there is no problem, return the address of the first byte of the allocated space on the heap. ( It calls malloc() or calloc() for space allocation). ;if the function is defined as: void *malloc_safe(Uint size); then malloc_safe(-5) will not have error messages. instead, 4294967291 byes will be allocated, causing a lot of confusion.
时间: 2023-02-06 11:12:33 浏览: 83
以下是用 C 语言实现上述要求的代码示例:
```
#include <stdio.h>
#include <stdlib.h>
void *malloc_safe(int size) {
if (size <= 0) {
printf("Error: size must be positive.\n");
exit(1);
}
void *ptr = malloc(size);
if (ptr == NULL) {
printf("Error: malloc failed.\n");
exit(1);
}
return ptr;
}
```
该代码中,我们先检查输入的 size 是否为正数,如果不是,就输出错误信息并退出程序。然后使用 malloc 函数分配内存,如果返回 NULL,则输出错误信息并退出程序。如果一切正常,则返回分配内存的首地址。
请注意,在使用 malloc_safe 函数分配内存后,调用者需要负责在使用完毕后调用 free 函数释放内存。
相关问题
帮我用c语言写出一段make a clone on the Heap for a piece of memory的代码,要求返回值为void*类型,The starting address of a memory is addr,The number of bytes of is size,并且帮我用上malloc_safe()函数
首先,我们需要包含头文件 `stdlib.h`,该头文件中包含了 `malloc` 和 `malloc_safe` 函数的声明。
然后,我们可以使用以下代码来实现在堆上克隆一段内存:
```c
void* make_clone_on_heap(void* addr, size_t size) {
// 使用 malloc_safe 分配内存
void* new_addr = malloc_safe(size);
// 复制内存
memcpy(new_addr, addr, size);
// 返回新内存块的起始地址
return new_addr;
}
```
其中,`malloc_safe` 函数是一个用于在堆上分配内存的安全版本的函数,它可以确保分配操作成功,否则会抛出异常。
请注意,这段代码中的 `memcpy` 函数用于将源内存块中的数据复制到目标内存块中。
最后,请记得在使用完新克隆的内存块后,使用 `free` 函数释放堆上的内存。
解释一下void *my_malloc(int size)
void *my_malloc(int size)是一个自定义的函数,其返回类型为void*,表示返回一个指向任意类型的指针。该函数的参数是一个整型变量size,用于指定所需分配内存的大小。
函数内部实现的具体细节可能因具体的代码而异,但通常的目标是在堆内存中分配一块指定大小的内存,并返回其起始地址。由于返回类型为void*,意味着函数可以返回任意类型的指针。
需要注意的是,使用该函数分配的内存需要手动释放,以避免内存泄漏。常用的释放内存的函数是free()。
相关推荐
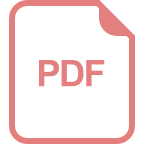
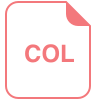
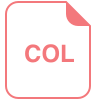
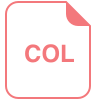
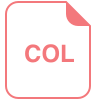
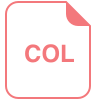







