如何用C语言写一个线程
时间: 2023-07-18 18:05:35 浏览: 94
在C语言中,可以使用标准库中的pthread库来创建和控制线程。下面是一个简单的示例程序,演示如何使用pthread库在C语言中创建和运行一个线程。
```c
#include <stdio.h>
#include <pthread.h>
void *my_thread_function(void *arg)
{
int i;
for (i = 0; i < 10; i++) {
printf("This is my thread function. i = %d\n", i);
}
return NULL;
}
int main()
{
pthread_t my_thread;
int result;
// 创建线程
result = pthread_create(&my_thread, NULL, my_thread_function, NULL);
if (result != 0) {
printf("Error creating thread\n");
return 1;
}
// 等待线程结束
result = pthread_join(my_thread, NULL);
if (result != 0) {
printf("Error joining thread\n");
return 1;
}
printf("Thread finished\n");
return 0;
}
```
在上面的示例程序中,我们定义了一个名为`my_thread_function`的函数作为线程的入口点。在`main`函数中,我们使用`pthread_create`函数创建了一个新的线程,并将`my_thread_function`函数作为线程的入口点。然后,我们使用`pthread_join`函数等待线程结束,并检查返回值以确保线程正常退出。
需要注意的是,创建线程时需要传递线程入口点函数的地址,而不是函数名。此外,在使用`pthread_create`函数时,还需要传递线程的属性参数和线程入口点函数的参数。在本例中,我们将线程的属性参数和线程入口点函数的参数都设置为NULL。
以上是一个简单的例子,实际使用中可能需要更多的线程控制和同步操作。您可以参考pthread库的文档,了解更多有关线程的函数和特性。
阅读全文
相关推荐
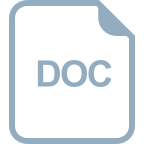
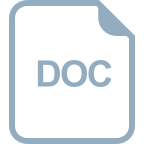
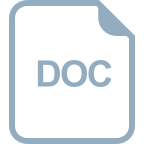


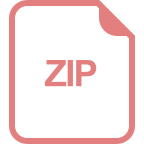
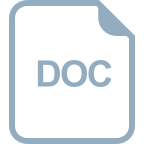
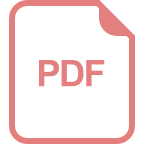
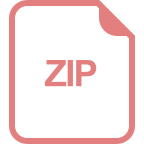
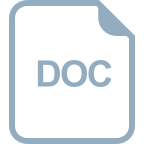
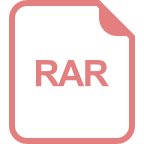
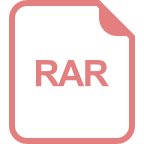





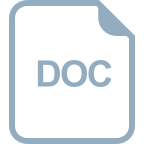