pta程序填空题以头插法建立单链表
时间: 2024-06-15 07:09:17 浏览: 160
PTA(Programming Test and Analysis)是一个在线编程练习平台,提供了各种编程题目供学生练习和测试。程序填空题是其中一种类型的题目,要求填写代码的空白部分以完成特定的功能。
头插法是一种常用的方法来建立单链表。它的基本思想是将新节点插入到链表的头部,使其成为新的头节点。下面是使用头插法建立单链表的示例代码:
```c++
#include <iostream>
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {}
};
ListNode* createLinkedList(int arr[], int n) {
ListNode* head = nullptr;
for (int i = 0; i < n; i++) {
ListNode* newNode = new ListNode(arr[i]);
newNode->next = head;
head = newNode;
}
return head;
}
void printLinkedList(ListNode* head) {
ListNode* cur = head;
while (cur != nullptr) {
std::cout << cur->val << " ";
cur = cur->next;
}
std::cout << std::endl;
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
ListNode* head = createLinkedList(arr, n);
printLinkedList(head);
return 0;
}
```
上述代码中,`createLinkedList`函数接受一个整型数组和数组长度作为参数,通过头插法建立一个单链表,并返回链表的头节点指针。`printLinkedList`函数用于打印链表的元素值。
阅读全文
相关推荐
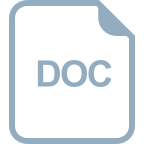
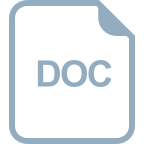
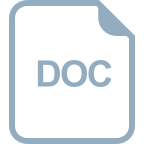
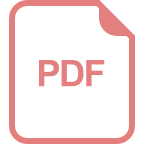














