贝叶斯神经网络预测的模型代码
时间: 2024-12-17 10:23:23 浏览: 2
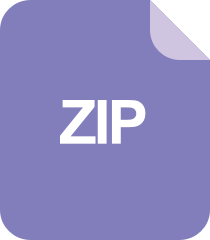
Bayesian_CNN_贝叶斯卷积神经网络_

贝叶斯神经网络(Bayesian Neural Networks, BNNs)是一种结合了概率论和神经网络的模型,它通过给每个权重分配一个先验分布,使得我们能够对模型的不确定性进行建模。在预测过程中,BNN不仅返回最可能的结果,还提供了一个关于这个结果的置信度。
以下是使用PyTorch库构建简单BNN的Python代码示例:
```python
import torch
from torch import nn, distributions
class BayesianLinear(nn.Module):
def __init__(self, in_features, out_features, prior_std=0.1):
super(BayesianLinear, self).__init__()
self.in_features = in_features
self.out_features = out_features
self.weight_mu = nn.Parameter(torch.Tensor(out_features, in_features).normal_(mean=0, std=prior_std))
self.weight_rho = nn.Parameter(torch.Tensor(out_features, in_features).fill_(0.5)) # 对数标准差
self.bias_mu = nn.Parameter(torch.Tensor(out_features).normal_(mean=0, std=prior_std))
self.bias_rho = nn.Parameter(torch.Tensor(out_features).fill_(0.5))
def forward(self, x):
weight_dist = distributions.Laplace(self.weight_mu, torch.exp(self.weight_rho))
bias_dist = distributions.Laplace(self.bias_mu, torch.exp(self.bias_rho))
linear_out = torch.matmul(x, weight_dist.sample()) + bias_dist.sample()
return linear_out
# 使用BNN层
model = nn.Sequential(
BayesianLinear(10, 20), # 输入10维特征,输出20维
BayesianLinear(20, 1) # 输出1维
)
# 预测时,需要一个样本输入并获取其后验分布
x = torch.randn(100, 10)
posterior_pred = model(x)
```
在这个例子中,我们创建了一个包含两个`BayesianLinear`层的网络。在每次前向传播时,权重和偏置都会从它们的后验分布中采样一次,从而得到一个随机的预测。
阅读全文
相关推荐
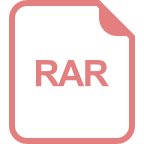
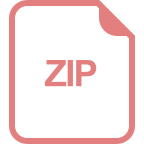
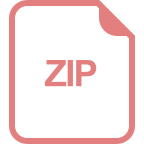
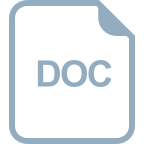
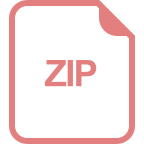
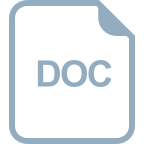
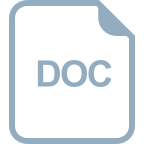
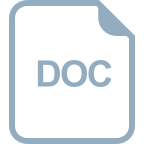
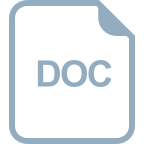
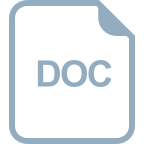
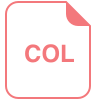





